JavaScript: Get a boolean determining if the passed value is an object or not
JavaScript fundamental (ES6 Syntax): Exercise-194 with Solution
Write a JavaScript program to get a boolean determining if the passed value is an object or not.
- Uses the Object constructor to create an object wrapper for the given value.
- If the value is null or undefined, create and return an empty object.
- Otherwise, return an object of a type that corresponds to the given value.
Sample Solution:
JavaScript Code:
// Define a function 'isObject' that checks if the given value 'obj' is an object
const isObject = obj =>
// Check if 'obj' is strictly equal to Object(obj)
obj === Object(obj);
// Test cases to check if the values are objects
console.log(isObject([1, 2, 3, 4]));
console.log(isObject([]));
console.log(isObject(['Hello!']));
console.log(isObject({ a: 1 }));
console.log(isObject({}));
console.log(isObject(true));
Output:
true true true true true false
Flowchart:
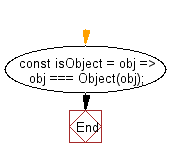
Live Demo:
See the Pen javascript-fundamental-exercise-194 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to check if a value is object-like. Check if the provided value is not null and its typeof is equal to 'object'.
Next: Write a JavaScript program to check if a given argument is a number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics