JavaScript: Check if a given argument is a number
JavaScript fundamental (ES6 Syntax): Exercise-195 with Solution
Check If Number
Write a JavaScript program to check if a given argument is a number.
- Use typeof to check if a value is classified as a number primitive.
- To safeguard against NaN, check if val === val (as NaN has a typeof equal to number and is the only value not equal to itself).
Sample Solution:
JavaScript Code:
// Define a function 'isNumber' that checks if the given value 'val' is a number
const isNumber = val =>
// Check if the type of 'val' is 'number'
typeof val === 'number';
// Test cases to check if the values are numbers
console.log(isNumber('1')); // false (the value is a string, not a number)
console.log(isNumber(1)); // true (the value is a number)
Output:
false true
Visual Presentation:
Flowchart:
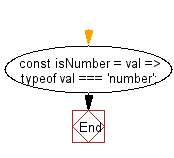
Live Demo:
See the Pen javascript-basic-exercise-195-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get a boolean determining if the passed value is an object or not.
Next: Write a JavaScript program that will return true if the specified value is null, false otherwise.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-195.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics