JavaScript: Generate a UUID in Node.JS.
JavaScript fundamental (ES6 Syntax): Exercise-254 with Solution
Generate UUID (Node.js)
Write a JavaScript program to generate a UUID in Node.JS. Use crypto API to generate a UUID, compliant with RFC4122 version 4.
- Use crypto.randomBytes() to generate a UUID, compliant with RFC4122 version 4.
- Use Number.prototype.toString(16) to convert it to a proper UUID.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Import the 'crypto' module from Node.js for cryptographic functionality
const crypto = require('crypto');
// Define a function 'UUIDGeneratorNode' to generate a UUID (Universally Unique Identifier) in a Node.js environment
const UUIDGeneratorNode = () =>
// Generate a UUID using a random number generator provided by the 'crypto' module
([1e7] + -1e3 + -4e3 + -8e3 + -1e11).replace(/[018]/g, c =>
// For each character 'c' in the UUID template, replace it with a randomly generated hexadecimal digit
(c ^ (crypto.randomBytes(1)[0] & (15 >> (c / 4)))).toString(16)
);
// Generate and log a UUID using the 'UUIDGeneratorNode' function
console.log(UUIDGeneratorNode());
Flowchart:
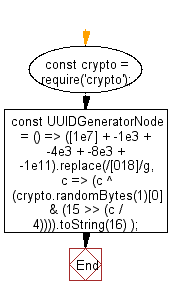
For more Practice: Solve these Related Problems:
- Write a JavaScript program in Node.js that uses the crypto module to generate an RFC4122 version 4 UUID.
- Write a Node.js function that creates a UUID by generating random bytes and formatting them into a standard string.
- Write a JavaScript program that leverages crypto.randomUUID() (if available) to produce a UUID and logs the result.
- Write a Node.js script that validates the generated UUID against the version 4 specification using regex.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to generate a UUID in a browser.
Next: Write a JavaScript program that will return true if the provided predicate function returns true for at least one element in a collection, false otherwise.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.