JavaScript: Generate a UUID in a browser
JavaScript fundamental (ES6 Syntax): Exercise-253 with Solution
Generate UUID (Browser)
Write a JavaScript program to generate a UUID in a browser.
- Use Crypto.getRandomValues() to generate a UUID, compliant with RFC4122 version 4.
- Use Number.prototype.toString(16) to convert it to a proper UUID.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'UUIDGeneratorBrowser' to generate a UUID (Universally Unique Identifier) in a web browser environment
const UUIDGeneratorBrowser = () =>
// Generate a UUID using a random number generator provided by the browser's crypto API
([1e7] + -1e3 + -4e3 + -8e3 + -1e11).replace(/[018]/g, c =>
// For each character 'c' in the UUID template, replace it with a randomly generated hexadecimal digit
(c ^ (crypto.getRandomValues(new Uint8Array(1))[0] & (15 >> (c / 4)))).toString(16)
);
// Generate and log a UUID using the 'UUIDGeneratorBrowser' function
console.log(UUIDGeneratorBrowser());
Output:
a47704c1-b9cb-481d-bada-bd6495a88ef7
Flowchart:
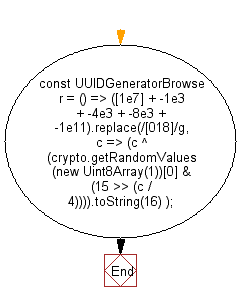
Live Demo:
See the Pen javascript-basic-exercise-253-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that generates a version 4 UUID using the browser’s crypto.getRandomValues() API.
- Write a JavaScript function that creates a UUID compliant with RFC4122 using random bytes from the browser.
- Write a JavaScript program that constructs a UUID string by replacing template placeholders with random hex digits.
- Write a JavaScript function that verifies the format of a generated UUID and logs whether it is valid.
Go to:
PREV : RGB to Color Code.
NEXT : Generate UUID (Node.js).
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.