JavaScript - Get the index of an array item in a for loop
JavaScript fundamental (ES6 Syntax): Exercise-268 with Solution
Index of Array Item in Loop
Write a JavaScript program to find the index of an array item in a for loop.
JavaScript's for...of loops provide an easy way to iterate over all kinds of iterables from arrays and stings to Map and Set objects. One supposed limitation over other options (e.g. Array.prototype.forEach()) is that you only get the value of each item in the iterable. But that is not necessarily the case, as you can easily leverage Array.prototype.entries() to get both the index and value of each array item:
Sample Solution:
JavaScript Code:
// Define an array 'colors' containing color names
const colors = ['Red', 'Green', 'Black', 'White'];
// Iterate over the array using the 'entries' method to get both index and value
for (let [index, item] of colors.entries()) {
// Print index and corresponding color name
console.log(`${index}: ${item}`);
}
Output:
0: Red 1: Green 2: Black 3: White
Flowchart:
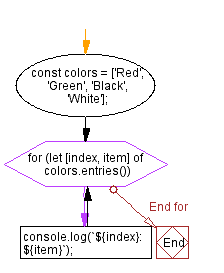
Live Demo:
See the Pen javascript-fundamental-exercise-268 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that finds the index of a specific item in an array using a traditional for loop.
- Write a JavaScript function that iterates over an array with a for loop and returns the index of the first occurrence of a target value.
- Write a JavaScript program that logs the index of each array element that meets a certain condition during a loop.
- Write a JavaScript function that demonstrates different looping constructs (for, while) to find an item’s index in an array.
Improve this sample solution and post your code through Disqus
Previous: Calculate the midpoint between two points.
Next: JavaScript functions exercises
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.