JavaScript: Calculate age
JavaScript Datetime: Exercise-18 with Solution
Calculate Age
Write a JavaScript program to calculate age.
Test Data:
console.log(calculate_age(new Date(1982, 11, 4)));
35
console.log(calculate_age(new Date(1962, 1, 1)));
56
Sample Solution:
JavaScript Code:
// Define a JavaScript function called calculate_age with parameter dob (date of birth)
function calculate_age(dob) {
// Calculate the difference in milliseconds between the current date and the provided date of birth
var diff_ms = Date.now() - dob.getTime();
// Create a new Date object representing the difference in milliseconds and store it in the variable age_dt (age Date object)
var age_dt = new Date(diff_ms);
// Calculate the absolute value of the difference in years between the age Date object and the year 1970 (UNIX epoch)
return Math.abs(age_dt.getUTCFullYear() - 1970);
}
// Output the calculated age for a person born on December 4, 1982
console.log(calculate_age(new Date(1982, 11, 4)));
// Output the calculated age for a person born on February 1, 1962
console.log(calculate_age(new Date(1962, 1, 1)));
Output:
35 56
Explanation:
In the exercise above,
- The code defines a JavaScript function named "calculate_age()" with one parameter 'dob', which represents the date of birth of a person.
- Inside the function:
- It calculates the difference in milliseconds between the current date (Date.now()) and the provided date of birth (dob.getTime()), and stores it in the variable 'diff_ms'.
- It creates a new Date object ('age_dt') representing the difference in milliseconds, essentially creating a date object that corresponds to the person's age.
- It calculates the absolute value of the difference in years between the year stored in the 'age_dt' object and the year 1970 (which is the UNIX epoch, used as a reference point).
- It returns the calculated age.
- The code then demonstrates the usage of the "calculate_age()" function by calling it with two different dates of birth:
- December 4, 1982 (new Date(1982, 11, 4))
- February 1, 1962 (new Date(1962, 1, 1))
Flowchart:
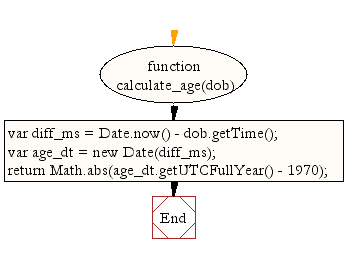
Live Demo:
See the Pen JavaScript - Calculate age-date-ex-18 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates age in years by subtracting the birth date from the current date.
- Write a JavaScript function that takes a Date object representing birthdate and returns the age by comparing year, month, and day.
- Write a JavaScript function that uses the difference in milliseconds between two dates to compute age.
- Write a JavaScript function that validates the birth date to ensure it is not in the future before calculating age.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to convert a Unix timestamp to time.
Next: Write a JavaScript function to get the day of the month, 2 digits with leading zeros.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.