JavaScript: Sort an array using Bubble Sorting method
JavaScript Object: Exercise-6 with Solution
Write a bubble sort algorithm in JavaScript.
Note: Bubble sort is a simple sorting algorithm that works by repeatedly stepping through the list to be sorted,
Sample Data: [6,4,0, 3,-2,1]
Expected Output : [-2, 0, 1, 3, 4, 6]
Sample Solution:
JavaScript Code:
Array.prototype.bubbleSort_algo = function()
{
var is_sorted = false;
while (!is_sorted)
{
is_sorted = true;
for (var n = 0; n < this.length - 1; n++)
{
if (this[n] > this[n+1]){
var x = this[n+1];
this[n+1] = this[n];
this[n] = x;
is_sorted = false;
}
}
}
return this;
};
console.log([6,4,0, 3,-2,1].bubbleSort_algo());
Output:
[-2,0,1,3,4,6]
Flowchart:
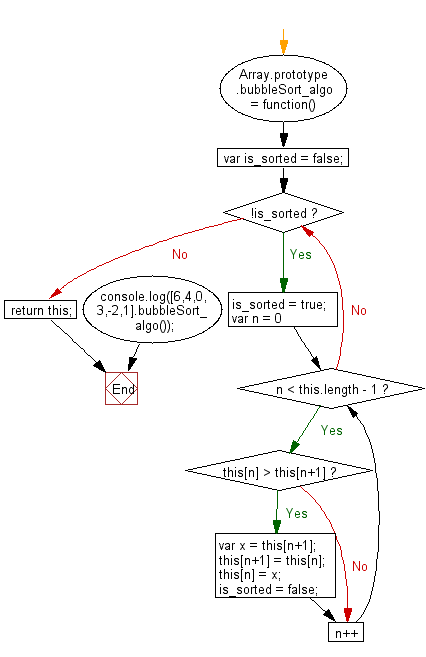
Live Demo:
See the Pen javascript-object-exercise-6 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to get the volume of a Cylinder with four decimal places using object classes.
Next: Write a JavaScript program which returns a subset of a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics