JavaScript: Calculate the volume of a Cylinder
JavaScript Object: Exercise-5 with Solution
Cylinder Volume
Write a JavaScript program to get the volume of a cylindrical with four decimal places using object classes.
Volume of a cylinder : V = πr2h
where r is the radius and h is the height of the cylinder.
Sample Solution:
JavaScript Code:
function Cylinder(cyl_height, cyl_diameter) {
this.cyl_height = cyl_height;
this.cyl_diameter = cyl_diameter;
}
Cylinder.prototype.Volume = function () {
var radius = this.cyl_diameter / 2;
return this.cyl_height * Math.PI * radius * radius;
};
var cyl = new Cylinder(7, 4);
// Volume of the cylinder with four decimal places.
console.log('volume = ' + cyl.Volume().toFixed(4));
Output:
volume = 87.9646
Flowchart:
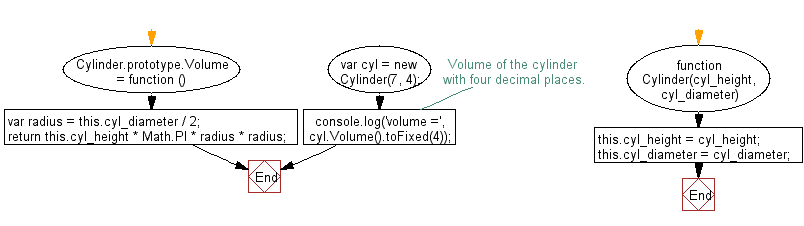
Live Demo:
See the Pen javascript-object-exercise-5 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript class for a cylinder that includes methods to calculate its volume and surface area.
- Write a JavaScript function that accepts radius and height as inputs and returns the cylinder volume rounded to four decimal places.
- Write a JavaScript function that validates input values for the radius and height before computing the volume.
- Write a JavaScript function that compares the volume of a cylinder with that of a cone having the same base and height.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to display the reading status (i.e. display book name, author name and reading status) of the following books.
Next: Write a Bubble Sort algorithm in JavaScript.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.