JavaScript: Rearrange a string to become a palindrome
JavaScript String: Exercise-57 with Solution
Rearrange to Palindrome
When a word, phrase, or sequence can be read both forward and backward, it is considered a palindrome. e.g., madam or nurses run.
Write a JavaScript function that receives a string and determines whether or not it can be rearranged to become a palindrome.
Test Data:
("maamd") -> true
("civic") -> true
("IO") -> false
(12321) -> "It must be a string."
Sample Solution:
JavaScript Code:
// Define a function named 'test' with a single parameter 'word'
const test = (word) => {
// Check if the input word is an empty string
if (word.length === 0)
{
// Return a message if the input string is empty
return 'String should not be empty!'
}
// Check if the input parameter 'word' is not a string
if (typeof word !== 'string')
{
// Return a message if the input is not a string
return 'It must be a string.'
}
// Use the spread operator to convert the string into an array of characters, then reduce it to count the occurrences of each character
const char_ctr = [...word].reduce((obj, el) => {
// If the character exists in the object, increment its count; otherwise, initialize it with a count of 1
obj[el] = obj[el] ? obj[el] + 1 : 1
return obj
}, {})
// Check if there are at most one character with an odd count (indicating the possibility of a palindrome)
return Object.values(char_ctr).filter(count => count % 2 !== 0).length <= 1
}
// Test the 'test' function with different input strings and output the result
console.log(test("maamd")) // true
console.log(test("civic")) // true
console.log(test("IO")) // false
console.log(test(12321)) // It must be a string.
Output:
true true false It must be a string.
Explanation:
In the exercise above,
- The function "test()" is defined using arrow function syntax, taking a single parameter named 'word'.
- It first checks if the input word is an empty string. If it is, the function returns a message indicating that the string should not be empty.
- Next, it checks if the input parameter 'word' is not a string using the 'typeof' operator. If it's not a string, the function returns a message indicating it must be a string.
- If the input is a non-empty string, the function counts the occurrences of each character in the word using the spread operator ([...word]) to convert the string into an array of characters. It then uses the "reduce()" method to count each character's occurrences.
- After counting the occurrences of each character, the function checks if there are at most one character with an odd count. If there is at most one character with an odd count, the function returns 'true', indicating that the word can form a palindrome. Otherwise, it returns 'false'.
- Finally, the function is tested with different input strings, and the results are logged to the console. If a 'non-string' input is provided, a message indicating so is logged instead of counting character occurrences.
Flowchart:
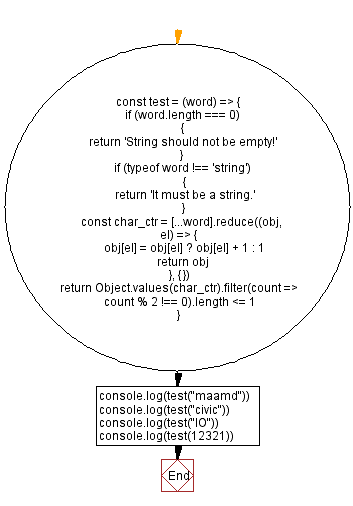
Live Demo:
See the Pen javascript-string-exercise-57 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if a string’s characters can be rearranged to form a palindrome using frequency counts.
- Write a JavaScript function that calculates the frequency of each character and determines if at most one odd count exists.
- Write a JavaScript function that returns true if the string can be rearranged into a palindrome, false otherwise.
- Write a JavaScript function that validates the input and ensures it is a string before processing for palindrome potential.
Improve this sample solution and post your code through Disqus.
Previous: Check a string is in Pascal case.
Next: Find the most frequent character in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.