PHP Exercises: Shift an element in left direction and return a new array
PHP Basic Algorithm: Exercise-124 with Solution
Write a PHP program to shift an element in left direction and return a new array.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($numbers)
{
// Calculate the size of the input array
$size = sizeof($numbers);
// Initialize an array to store the shifted numbers
$shiftNums = [$size];
// Iterate through the elements of the input array using a for loop
for ($i = 0; $i < $size; $i++)
{
// Shift the numbers to the left by one position, considering the circular nature of the shift
$shiftNums[$i] = $numbers[($i + 1) % $size];
}
// Return the array of shifted numbers
return $shiftNums;
}
// Call the 'test' function with an example array and store the result in the variable 'result'
$result = test([10, 20, -30, -40, 50] );
// Print the result array as a string
echo "New array: " . implode(',', $result);
?>
Sample Output:
New array: 20,-30,-40,50,10
Flowchart:
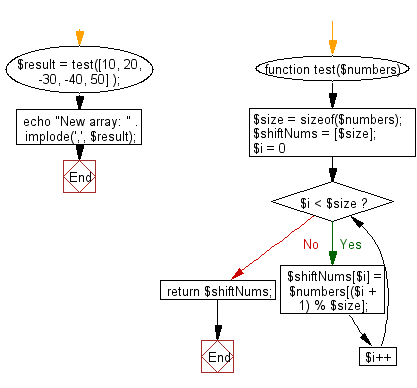
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check a given array of integers and return true if the array contains three increasing adjacent numbers.
Next: Write a PHP program to create a new array taking the elements before the element value 5 from a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics