PHP Exercises: Check a given array of integers and return true if the array contains three increasing adjacent numbers
123. Contains Three Increasing Adjacent Numbers
Write a PHP program to check a given array of integers and return true if the array contains three increasing adjacent numbers.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($numbers)
{
// Iterate through the elements of the array using a for loop
for ($i = 0; $i <= sizeof($numbers) - 3; $i++)
{
// Check if the current element is equal to the next element minus 1
// and if the current element is equal to the element after the next element minus 2
if ($numbers[$i] == $numbers[$i + 1] - 1 && $numbers[$i] == $numbers[$i + 2] - 2)
{
// Return true if the condition is met
return true;
}
}
// Return false if the condition is not met for any set of three consecutive elements
return false;
}
// Use 'var_dump' to print the result of calling 'test' with different arrays
var_dump(test([1, 2, 3, 5, 3, 7]));
var_dump(test([3, 7, 5, 5, 3, 7]));
var_dump(test([3, 7, 5, 5, 6, 7, 5]));
?>
Sample Output:
bool(true) bool(false) bool(true)
Flowchart:
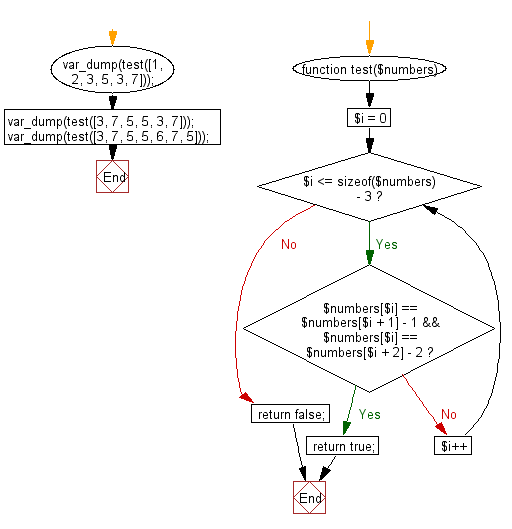
For more Practice: Solve these Related Problems:
- Write a PHP script to detect whether an array contains any three consecutive increasing numbers.
- Write a PHP function to iterate over an array and return true if a subsequence of three elements is strictly increasing.
- Write a PHP program to use a sliding window over an array and check if each trio is in ascending order.
- Write a PHP script to implement logic that compares consecutive triplets in an array to determine if an increasing pattern exists.
Go to:
PREV : Same Number of Elements at Start and End.
NEXT : Shift Array Elements Left.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.