PHP Exercises: Check a given array of integers and return true if the specified number of same elements appears at the start and end of the given array
122. Same Number of Elements at Start and End
Write a PHP program to check a given array of integers and return true if the specified number of same elements appears at the start and end of the given array.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers and an integer length as parameters
function test($numbers, $len)
{
// Calculate the size of the array
$arra_size = sizeof($numbers);
// Iterate through the elements of the array up to the specified length
for ($i = 0; $i < $len; $i++)
{
// Check if the current element is not equal to the corresponding element from the end of the array
if ($numbers[$i] != $numbers[$arra_size - $len + $i])
{
// Return false if the condition is met
return false;
}
}
// Return true if the specified length of elements from the beginning and end of the array are equal
return true;
}
// Use 'var_dump' to print the result of calling 'test' with different arrays and lengths
var_dump(test([3, 7, 5, 5, 3, 7], 2));
var_dump(test([3, 7, 5, 5, 3, 7], 3));
var_dump(test([3, 7, 5, 5, 3, 7, 5], 3));
Sample Output:
bool(true) bool(false) bool(true)
Flowchart:
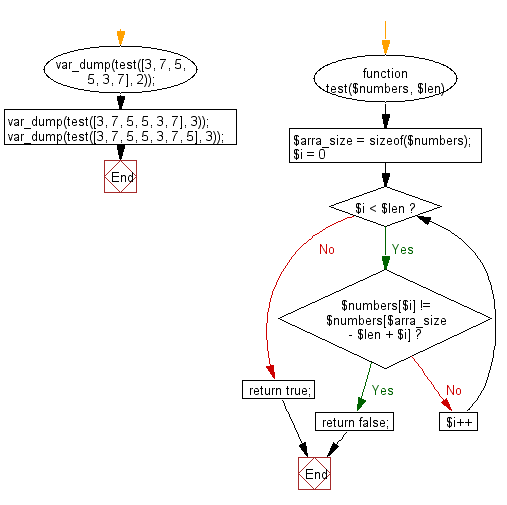
For more Practice: Solve these Related Problems:
- Write a PHP script to check if an array contains the same number of repeating elements at the beginning and the end.
- Write a PHP function that compares the first n and last n elements of an array and returns true if they are identical.
- Write a PHP program to extract the first and last segments of an array and compare them element by element.
- Write a PHP script to verify that an array’s starting and ending sequences of a specified length are the same.
Go to:
PREV : Check Every 5 is Paired with Another.
NEXT : Contains Three Increasing Adjacent Numbers.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.