PHP Exercises: Check if a given string contains between 2 and 4 'z' character
PHP Basic Algorithm: Exercise-22 with Solution
Write a PHP program to check if a given string contains between 2 and 4 'z' character.
Sample Solution:
PHP Code :
<?php
// Define a function that checks if a string contains the character 'z' 2 to 3 times
function test($s)
{
// Initialize a counter variable
$ctr = 0;
// Loop through each character in the string
for ($i = 0; $i < strlen($s); $i++)
{
// Check if the current character is 'z' and increment the counter if true
if (substr($s, $i, 1) == 'z')
{
$ctr++;
}
}
// Return true if the counter is greater than 1 and less than 4, indicating 'z' occurs 2 to 3 times
return $ctr > 1 && $ctr < 4;
}
// Test the function with different strings
var_dump(test("frizz"));
var_dump(test("zane"));
var_dump(test("Zazz"));
var_dump(test("false"));
?>
Sample Output:
bool(true) bool(false) bool(true) bool(false)
Visual Presentation:
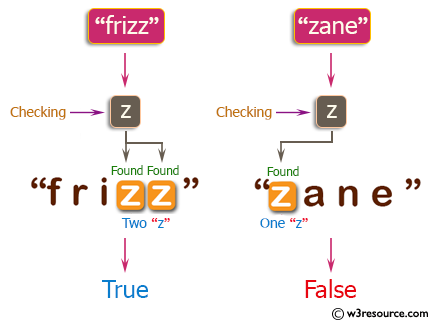
Flowchart:
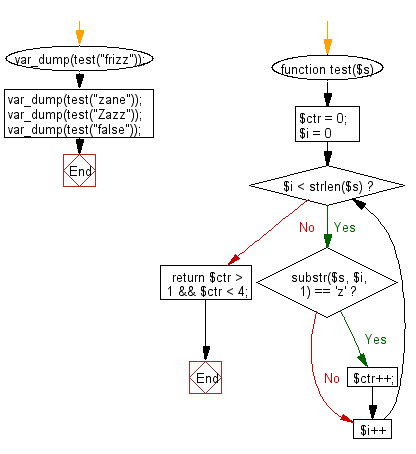
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to find the larger value from two positive integer values that is in the range 20..30 inclusive, or return 0 if neither is in that range.
Next: Write a PHP program to check if two given non-negative integers have the same last digit.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics