PHP Exercises: Find the larger value from two positive integer values that is in the range 20..30 inclusive
21. Largest in Range 20..30
Write a PHP program to find the larger value from two positive integer values that is in the range 20..30 inclusive, or return 0 if neither is in that range.
Sample Solution:
PHP Code :
<?php
// Define a function that compares two values based on certain conditions
function test($x, $y)
{
// Check if both $x and $y are within the range of 20 to 30
if ($x >= 20 && $x <= 30 && $y >= 20 && $y <= 30) {
// Compare $x and $y, return the greater value
if ($x >= $y) {
return $x;
} else {
return $y;
}
} elseif ($x >= 20 && $y <= 30) {
// Check if only $x is within the range, return $x
return $x;
} elseif ($y >= 20 && $y <= 30) {
// Check if only $y is within the range, return $y
return $y;
} else {
// Return 0 if neither condition is met
return 0;
}
}
// Test the function with different sets of coordinates
echo test(78, 95)."\n";
echo test(20, 30)."\n";
echo test(21, 25)."\n";
echo test(28, 28)."\n";
?>
Explanation:
- Function Definition:
- The test function takes two parameters, $x and $y, and compares them based on specific conditions.
- Condition Checks:
- Both values within the range [20, 30]:
- If both $x and $y are between 20 and 30, the function compares them and returns the greater value.
- Only $x within the range [20, 30]:
- If only $x is within the range, the function returns $x.
- Only $y within the range [20, 30]:
- If only $y is within the range, the function returns $y.
- Neither value in the range:
- If neither $x nor $y is within the range, the function returns 0.
- Function Calls and Results:
- First Call: test(78, 95) returns 0 since neither value is within the range.
- Second Call: test(20, 30) returns 30 as both are in range, and 30 is the greater value.
- Third Call: test(21, 25) returns 25 as both are in range, and 25 is the greater value.
- Fourth Call: test(28, 28) returns 28 as both are in range, and both values are equal.
Output:
0 30 25 28
Visual Presentation:
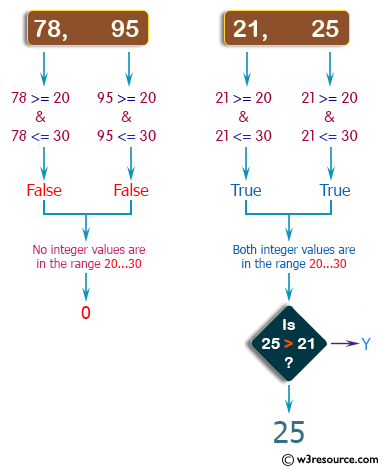
Flowchart:
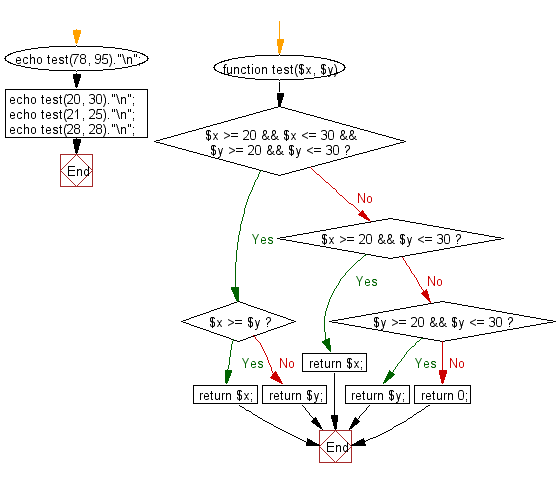
For more Practice: Solve these Related Problems:
- Write a PHP script to compare two positive integers and return the larger one if it falls within 20–30, otherwise return 0.
- Write a PHP function to check if either of two numbers is in the range 20 to 30 and then pick the maximum value.
- Write a PHP program to evaluate two numbers for range membership and output the largest if qualified or zero if not.
- Write a PHP script to integrate conditional operators to select the greater number from two inputs only if they are within the desired range.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check which number nearest to the value 100 among two given integers. Return 0 if the two numbers are equal.
Next: Write a PHP program to check if a given string contains between 2 and 4 'z' character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.