PHP Exercises: Sort a collection of given arrays or objects by key
PHP: Exercise-93 with Solution
Write a PHP program to sort a collection of given arrays or objects by key.
Sample Solution:
PHP Code:
<?php
// Licence: https://bit.ly/2CFA5XY
// Function definition for 'orderBy' that takes an array of items, an attribute, and an order ('asc' or 'desc') as parameters
function orderBy($items, $attr, $order)
{
// Initialize an empty associative array to store the items with keys based on the specified attribute
$sortedItems = [];
// Iterate through each item in the array
foreach ($items as $item) {
// Determine the key based on whether the item is an object or an array
$key = is_object($item) ? $item->{$attr} : $item[$attr];
// Assign the item to the associative array using the calculated key
$sortedItems[$key] = $item;
}
// Check if the order is 'desc' and use 'krsort' to sort the array in descending order based on keys
// If the order is not 'desc', use 'ksort' to sort the array in ascending order based on keys
if ($order === 'desc') {
krsort($sortedItems);
} else {
ksort($sortedItems);
}
// Use 'array_values' to reindex the array after sorting
return array_values($sortedItems);
}
// Call 'orderBy' with an array of associative arrays, an attribute ('id'), and an order ('desc')
// Display the result using 'print_r'
print_r(orderBy(
[
['id' => 2, 'name' => 'Red'],
['id' => 3, 'name' => 'Black'],
['id' => 1, 'name' => 'Green']
],
'id',
'desc'
));
?>
Sample Output:
Array ( [0] => Array ( [id] => 3 [name] => Black ) [1] => Array ( [id] => 2 [name] => Red ) [2] => Array ( [id] => 1 [name] => Green ) )
Flowchart:
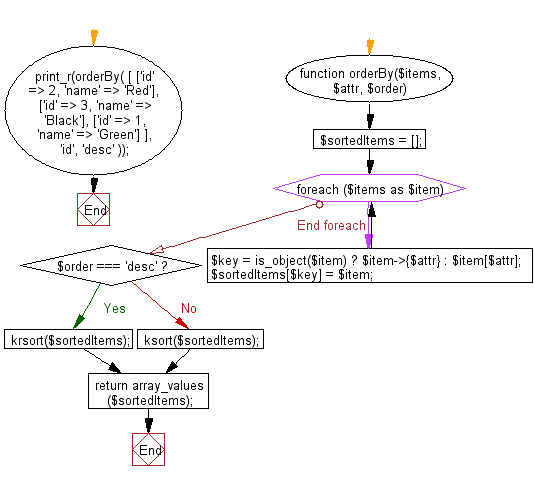
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to filter out the elements of an given array, that have one of the specified values.
Next: Write a PHP program to check if two numbers are approximately equal to each other.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics