PHP Exercises: Check if two numbers are approximately equal to each other
PHP: Exercise-94 with Solution
Write a PHP program to check if two numbers are approximately equal to each other.
Note: Use abs() to compare the absolute difference of the two values to epsilon. Omit the third parameter, epsilon, to use a default value of 0.001.
Sample Solution:
PHP Code:
<?php
// Licence: https://bit.ly/2CFA5XY
// Function definition for 'approximatelyEqual' that takes two numbers and an optional epsilon value as parameters
function approximatelyEqual($number1, $number2, $epsilon = 0.001)
{
// Check if the absolute difference between the numbers is less than the epsilon value
if (abs($number1 - $number2) < $epsilon)
// If approximately equal, return 1 (true)
return 1;
else
// If not approximately equal, return 0 (false)
return 0;
}
// Call 'approximatelyEqual' with two numbers and display the result using 'print_r'
print_r(approximatelyEqual(10.0, 10.00001));
// Display a newline
echo("\n");
// Call 'approximatelyEqual' with two different numbers and display the result using 'print_r'
print_r(approximatelyEqual(10.0, 10.01));
?>
Explanation:
- Function Definition:
- The approximatelyEqual function takes three parameters:
- $number1: the first number to compare.
- $number2: the second number to compare.
- $epsilon: an optional precision threshold, defaulted to 0.001.
- Check Approximate Equality:
- The function calculates the absolute difference between $number1 and $number2 using abs($number1 - $number2).
- If this difference is less than $epsilon, the numbers are considered approximately equal.
- Return Result:
- If the numbers are approximately equal, the function returns 1 (true).
- If not, it returns 0 (false).
- Function Calls and Output:
- approximatelyEqual(10.0, 10.00001) checks if 10.0 and 10.00001 are approximately equal within 0.001. Result: 1 (true).
- approximatelyEqual(10.0, 10.01) checks if 10.0 and 10.01 are approximately equal within 0.001. Result: 0 (false).
Output:
1 0
Flowchart:
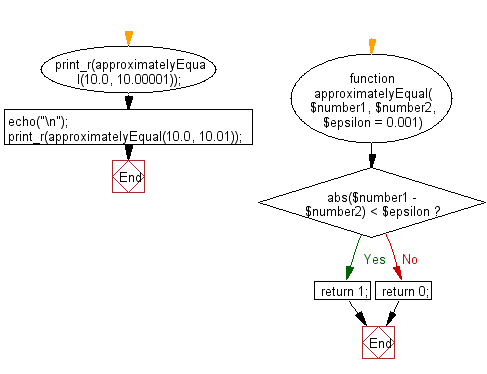
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to sort a collection of given arrays or objects by key.
Next: Write a PHP program to check if a given string starts with a given substring.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/php-exercises/php-basic-exercise-94.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics