PHP Exercises: Check if a given string starts with a given substring
95. Check String Starts With Substring
Write a PHP program to check if a given string starts with a given substring.
Sample Solution:
PHP Code:
<?php
// Licence: https://bit.ly/2CFA5XY
// Function definition for 'startsWith' that takes a haystack string and a needle string as parameters
function startsWith($haystack, $needle)
{
// Use 'strpos' to find the position of the needle in the haystack
// Check if the position is at the beginning (index 0) of the haystack
return strpos($haystack, $needle) === 0;
}
// Call 'startsWith' with a haystack string and a needle string, then display the result using 'print_r'
print_r(startsWith('Hi, this is me', 'Hi'));
?>
Explanation:
- Function Definition:
- The startsWith function takes two parameters:
- $haystack: the main string in which to search.
- $needle: the substring to check if it appears at the beginning of $haystack.
- Check Start Position:
- The function uses strpos($haystack, $needle) to find the position of $needle within $haystack.
- It then checks if $needle is found at the very beginning (position 0) of $haystack.
- Return Result:
- If $needle is at the start of $haystack, the function returns true.
- Otherwise, it returns false.
- Function Call and Output:
- startsWith('Hi, this is me', 'Hi') checks if 'Hi' is at the beginning of 'Hi, this is me'. Result: true (output as 1 with print_r).
Output:
1
Flowchart:
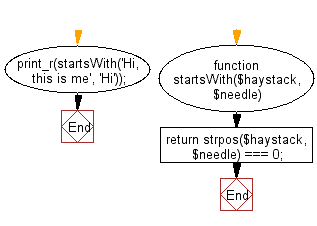
For more Practice: Solve these Related Problems:
- Write a PHP script to determine if a given string starts with a specific substring using substr comparison.
- Write a PHP function to check whether the first few characters of a string match a target prefix.
- Write a PHP script to use strpos or regex to verify if a string begins with the specified sequence.
- Write a PHP script to return a boolean indicating whether the initial segment of a string equals a given substring.
Go to:
PREV : Approximately Equal Number Check.
NEXT : Count Vowels in a String.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.