PHP Exercises: Count number of vowels in a given string
96. Count Vowels in a String
Write a PHP program to count number of vowels in a given string.
Note: Use a regular expression to count the number of vowels (A, E, I, O, U) in a string.
Sample Solution:
PHP Code:
<?php
// Licence: https://bit.ly/2CFA5XY
// Function definition for 'count_Vowels' that takes a string as a parameter
function count_Vowels($string)
{
// Use 'preg_match_all' with a regular expression to find all vowels (case-insensitive) in the string
preg_match_all('/[aeiou]/i', $string, $matches);
// Return the count of matched vowels found in the string
return count($matches[0]);
}
// Call 'count_Vowels' with a string and display the result using 'print_r'
print_r(count_Vowels('sampleInput'));
?>
Explanation:
- Function Definition:
- The count_Vowels function takes a single parameter:
- $string: the input string in which to count vowels.
- Finding Vowels:
- The function uses preg_match_all with the regular expression /[aeiou]/i:
- [aeiou]: matches any vowel (a, e, i, o, u).
- /i: makes the matching case-insensitive, so it includes both uppercase and lowercase vowels.
- The matching vowels are stored in $matches.
- Count Matched Vowels:
- count($matches[0]) returns the number of vowels found in $string.
- Function Call and Output:
- count_Vowels('sampleInput') counts the vowels in 'sampleInput' and outputs the result using print_r.
Output:
4
Flowchart:
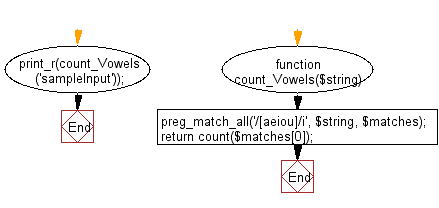
For more Practice: Solve these Related Problems:
- Write a PHP script to count the number of vowels in a string using regular expressions with case insensitivity.
- Write a PHP function to iterate over each character in a string and tally vowels found.
- Write a PHP script to compute the total number of vowels in an input sentence by filtering characters.
- Write a PHP script to use preg_match_all to count occurrences of A, E, I, O, U in a provided string.
Go to:
PREV : Check String Starts With Substring.
NEXT : Decapitalize the First Letter.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.