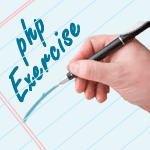
1. Write a PHP script to : - Go to the editor
a) transform a string all uppercase letters.
b) transform a string all lowercase letters.
c) make a string's first character uppercase.
d) make a string's first character of all the words uppercase.
<?php //all uppercase letters print(strtoupper("the quick brown fox jumps over the lazy dog.<br>")); //all lowercase letters print(strtolower("THE QUICK BROWN FOX JUMPS OVER THE LAZY DOG<br>")); // make a string's first character uppercase print(ucfirst("the quick brown fox jumps over the lazy dog.<br>")); // make a string's first character of all the words uppercase print(ucwords("the quick brown fox jumps over the lazy dog.<br>")); ?>
2. Write a PHP script to split the following string. Go to the editor
Sample string : '082307'
Expected Output : 08:23:07
<?php $str1= '082307'; echo substr(chunk_split($str1, 2, ':'), 0, -1); ?>
3. Write a PHP script to check if a string contains specific string? Go to the editor
Sample string : 'The quick brown fox jumps over the lazy dog.'
Check whether the said string contains the string 'jumps'.
<?php $str1 = 'The quick brown fox jumps over the lazy dog.'; if (strpos($str1,'jumps') !== false) { echo 'The specific word is present.'; } else { echo 'The specific word is not present.'; } ?>
4. Write a PHP script to convert the value of a PHP variable to string. Go to the editor
<?php $x = 20; // $x is an integer $str1 = (string)$x; // $str1 is a string now // Check whether $x and $str1 are equal or not if ($x === $str1) { echo "They are the same"; } else { echo "They are not same"; } ?>
5.Write a PHP script to extract the file name from the following string. Go to the editor
Sample String : 'www.example.com/public_html/index.php'
Expected Output : 'index.php'
<?php $path = 'www.example.com/public_html/index.php'; $file_name = substr(strrchr($path, "/"), 1); echo $file_name; // "index.php" ?>
6. Write a PHP script to extract the user name from the following email ID. Go to the editor
Sample String : '[email protected]'
Expected Output : 'rayy'
<?php $mailid = '[email protected]'; $user = strstr($mailid, '@', true); echo $user; ?>
7. Write a PHP script to get the last three characters of a string. Go to the editor
Sample String : '[email protected]'
Expected Output : 'com'
<?php $str1 = '[email protected]'; echo substr($str1, -3); ?>
8. Write a PHP script to format values in currency style. Go to the editor
Sample values : value1 = 65.45, value2 = 104.35
Expected Result : 169.80
<?php $value1 = 65.45; $value2 = 104.35; echo sprintf("%01.2f", $value1+$value2); ?>
9. Write a PHP script to generate simple random password [do not use rand() function] from a given string. Go to the editor
Sample string : '1234567890ABCDEFGHIJKLMNOPQRSTUVWXYZabcefghijklmnopqrstuvwxyz'
Note : Password length may be 6, 7, 8 etc.
<?php function password_generate($chars) { $data = '1234567890ABCDEFGHIJKLMNOPQRSTUVWXYZabcefghijklmnopqrstuvwxyz'; return substr(str_shuffle($data), 0, $chars); } echo password_generate(7); ?>
10. Write a PHP script to replace the first 'the' of the following string with 'That'. Go to the editor
Sample date : 'the quick brown fox jumps over the lazy dog.'
Expected Result : That quick brown fox jumps over the lazy dog.
<?php $str = 'the quick brown fox jumps over the lazy dog.'; echo preg_replace('/the/', 'That', $str, 1); ?>
11. Write a PHP script to find first character that is different between two strings. Go to the editor
String1 : 'football'
String2 : 'footboll'
Expected Result : First difference between two strings at position 5: "a" vs "o"
<?php $str1 = 'football'; $str2 = 'footboll'; $str_pos = strspn($str1 ^ $str2, "\0"); printf('First difference between two strings at position %d: "%s" vs "%s"', $str_pos, $str1[$str_pos], $str2[$str_pos]); ?>
12. Write a PHP script to put a string in an array. Go to the editor
Sample strings : "Twinkle, twinkle, little star,\nHow I wonder what you are.\nUp above the world so high,\nLike a diamond in the sky.";
Expected Result (using var_dump()) : array(4) { [0]=> string(30) "Twinkle, twinkle, little star," [1]=> string(26) "How I wonder what you are." [2]=> string(27) "Up above the world so high," [3]=> string(26) "Like a diamond in the sky." }
<?php $str = "Twinkle, twinkle, little star,\nHow I wonder what you are.\nUp above the world so high,\nLike a diamond in the sky."; $arra1 = explode("\n", $str); var_dump($arra1); ?>
13. Write a PHP script to get the filename component of the following path. Go to the editor
Sample path : "http://www.w3resource.com/index.php"
Expected Output : 'index'
<?php $path = 'www.example.com/public_html/index.php'; $file = basename($path, ".php"); echo $file; ?>
14. Write a PHP script to print the next character. Go to the editor
Sample character : 'a'
Expected Output : 'b'
Sample character : 'z'
Expected Output : 'a'
<?php $cha = 'a'; $next_cha = ++$cha; //The following if condition prevent you to go beyond 'z' or 'Z' and will reset to 'a' or 'A'. if (strlen($next_cha) > 1) { $next_cha = $next_cha[0]; } echo $next_cha; ?>
15. Write a PHP script to remove a part of a string from the beginning. Go to the editor
Sample string : '[email protected]'
Expected Output : 'example.com'
<?php $sub_string = 'rayy@'; $str = '[email protected]'; if (substr($str, 0, strlen($sub_string)) == $sub_string) { $str = substr($str, strlen($sub_string)); } echo $str; ?>
16. Write a PHP script to get a hex dump of a string ? Go to the editor
Sample string : '[email protected]'
<?php $str = '[email protected]'; echo bin2hex($str); ?>
17. Write a PHP script to insert a string at the specified position in a given string. Go to the editor
Original String : 'The brown fox'
Insert 'quick' between 'The' and 'brown'.
Expected Output : 'The quick brown fox'
<?php $original_string = 'The brown fox'; $string_to_insert ='quick'; $insert_pos = 4; $new_string = substr_replace($original_string, $string_to_insert.' ', $insert_pos, 0); echo $new_string; ?>
18. Write a PHP script to get the first word of a sentence. Go to the editor
Original String : 'The quick brown fox'
Expected Output : 'The'
<?php $s = 'The quick brown fox'; $arr1 = explode(' ',trim($s)); echo $arr1[0]; ?>
19. Write a PHP script to remove all leading zeroes from a string. Go to the editor
Original String : '000547023.24'
Expected Output : '547023.24'
<?php $x = '000547023.24'; $str1 = ltrim($x, '0'); echo $str1; ?>
20. Write a PHP script to remove part of a string. Go to the editor
Original String : 'The quick brown fox jumps over the lazy dog'
Remove 'fox' from the above string.
Expected Output : 'The quick brown jumps over the lazy dog'
<?php $my_str = 'The quick brown fox jumps over the lazy dog'; echo str_replace("fox", "", $my_str); ?>
21. Write a PHP script to remove trailing slash from a string. Go to the editor
Original String : 'The quick brown fox jumps over the lazy dog///'
Expected Output : 'The quick brown fox jumps over the lazy dog'
<?php $my_str = 'The quick brown fox jumps over the lazy dog///'; echo rtrim($my_str, '/'); ?>
22. Write a PHP script to get the characters after the last '/' in an url. Go to the editor
Sample URL : 'http://www.example.com/5478631'
Expected Output : '5478631'
<?php $my_url = 'http://www.example.com/5478631'; echo substr($my_url, strrpos($my_url, '/' )+1); ?>
23. Write a PHP script to replace multiple characters from the following string. Go to the editor
Sample String : '\"\1+2/3*2:2-3/4*3'
Expected Output : '1 2 3 2 2 3 4 3'
<?php $my_str = '\"\1+2/3*2:2-3/4*3'; echo str_replace(str_split('\\/:*?"<>|+-'), ' ', $my_str); ?>
24. Write a PHP script to select first 5 words from the following string. Go to the editor
Sample String : 'The quick brown fox jumps over the lazy dog'
Expected Output : 'The quick brown fox jumps'
<?php $my_string = 'The quick brown fox jumps over the lazy dog'; echo implode(' ', array_slice(explode(' ', $my_string), 0, 5)); ?>
25. Write a PHP script to remove comma(s) from the following numeric string. Go to the editor
Sample String : '2,543.12'
Expected Output : 2543.12
<?php $str1 = "2,543.12"; $x = str_replace( ',', '', $str1); if( is_numeric($x)) { echo $x; } ?>
26. Write a PHP script to print letters from 'a' to 'z'.Go to the editor
Expected Result : abcdefghijklmnopqrstuvwxyz
<?php for ($x = ord('a'); $x <= ord('z'); $x++) echo chr($x); ?>
More to Come !