Python: Create a new string with no duplicate consecutive letters from a given string
Remove Consecutive Duplicates
Write a Python program to create a string with no duplicate consecutive letters from a given string.
Sample Solution:
Python Code:
# Define a function named no_consecutive_letters that takes a string (txt) as an argument.
def no_consecutive_letters(txt):
# Return the first character of the string and join the characters where the current character is not equal to the previous character.
# The expression txt[i] for i in range(1, len(txt)) if txt[i] != txt[i-1] iterates over the characters, excluding consecutive duplicates.
return txt[0] + ''.join(txt[i] for i in range(1, len(txt)) if txt[i] != txt[i-1])
# Test the function with different strings and print the results.
# Test case 1
print(no_consecutive_letters("PPYYYTTHON"))
# Test case 2
print(no_consecutive_letters("PPyyythonnn"))
# Test case 3
print(no_consecutive_letters("Java"))
# Test case 4
print(no_consecutive_letters("PPPHHHPPP"))
Sample Output:
PYTHON Python Java PHP
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named "no_consecutive_letters()" that takes a string (txt) as an argument.
- Return Statement:
- The function returns the first character of the string concatenated with the characters where the current character is not equal to the previous character.
- Joining characters:
- The "join()" method is used to concatenate characters where consecutive duplicates are excluded.
Pictorial Presentation:
Flowchart:
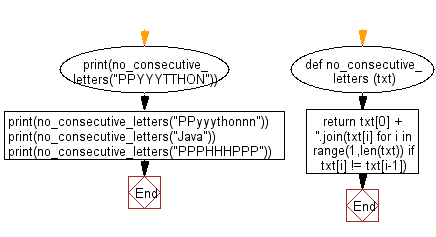
For more Practice: Solve these Related Problems:
- Write a Python program to remove consecutive duplicate characters from a string while preserving letter case.
- Write a Python program to compress a string by replacing groups of consecutive duplicate letters with a single instance.
- Write a Python program to iteratively remove consecutive duplicates from a string until no such duplicates remain.
- Write a Python program to remove only consecutive duplicate vowels from a given string.
Go to:
Previous: Write a Python program to find the name of the oldest student from a given dictionary containing the names and ages of a group of students.
Next: Write a Python program to check whether two given lines are parallel or not.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.