Python: Show the individual process IDs involved
Python Basic - 1: Exercise-118 with Solution
In multiprocessing, processes are spawned by creating a Process object.
Write a Python program to show the individual process IDs (parent process, process ID etc.) involved.
Sample Solution:
Python Code:
# Import the 'Process' class from the 'multiprocessing' module.
from multiprocessing import Process
# Import the 'os' module for interacting with the operating system.
import os
# Define a function 'info' to print process-related information.
def info(title):
print(title)
print('module name:', __name__)
print('parent process:', os.getppid())
print('process id:', os.getpid())
# Define a function 'f' that takes a name as an argument.
def f(name):
# Call the 'info' function to print process-related information.
info('function f')
# Print a greeting using the provided name.
print('hello', name)
# Check if the script is being run as the main program.
if __name__ == '__main__':
# Call the 'info' function to print process-related information for the main process.
info('Main line')
# Create a new 'Process' object, specifying the target function 'f' and its arguments.
p = Process(target=f, args=('bob',))
# Start the new process.
p.start()
# Wait for the child process to complete before continuing.
p.join()
Sample Output:
Main line module name: __main__ parent process: 23967 process id: 27986 function f module name: __main__ parent process: 27986 process id: 27987 hello bob
Explanation:
Here is a breakdown of the above Python code:
- Module Imports:
- The code imports the 'Process' class from the 'multiprocessing' module for working with processes.
- The 'os' module is imported for interacting with the operating system.
- Function definitions:
- The 'info' function prints information about the module name, parent process ID, and process ID.
- The 'f' function prints a greeting using the provided name.
- Main Program:
- The script checks if it is being run as the main program using if name == '__main__':.
- Process creation and execution:
- A new 'Process' object is created, specifying the target function 'f' and its arguments.
- The child process is started using p.start().
- The script waits for the child process to complete before continuing using p.join().
Flowchart:
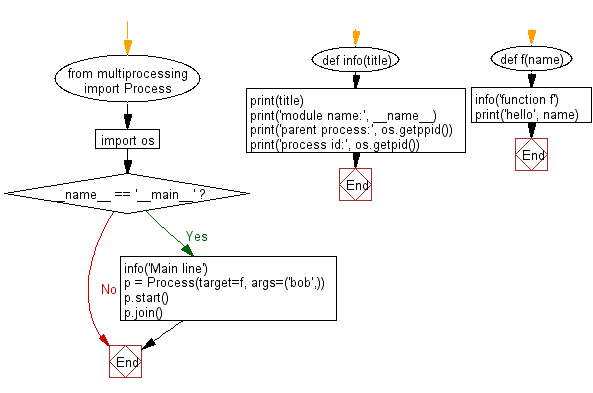
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to make a request to a web page, and test the status code, also display the html code of the specified web page.
Next: Write a Python program to check if two given numbers are Co Prime or not. Return True if two numbers are Co Prime otherwise return false.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics