Python: Compute the sum of the negative and positive numbers of an array of integers and display the largest sum
Python Basic - 1: Exercise-133 with Solution
Write a Python program to compute the sum of the negative and positive numbers in an array of integers and display the largest sum.
Sample Solution
Python Code:
# Define a function 'test' that takes a list of numbers as input and returns the largest sum
# among positive and negative numbers separately.
def test(lst):
# Initialize variables to store the sum of positive and negative numbers.
pos_sum = 0
neg_sum = 0
# Iterate through each number in the input list.
for n in lst:
# Check if the number is positive.
if n > 0:
pos_sum += n # Add the positive number to the positive sum.
# Check if the number is negative.
elif n < 0:
neg_sum += n # Add the negative number to the negative sum.
# Return the largest sum among positive and negative numbers using the 'max' function,
# with 'key=abs' to consider the absolute values.
return max(pos_sum, neg_sum, key=abs)
# Test cases with different sets of numbers.
nums = {0, -10, -11, -12, -13, -14, 15, 16, 17, 18, 19, 20}
print("Original array elements:")
print(nums)
print("Largest sum - Positive/Negative numbers of the said array: ", test(nums))
nums = {-11, -22, -44, 0, 3, 4, 5, 9}
print("\nOriginal array elements:")
print(nums)
print("Largest sum - Positive/Negative numbers of the said array: ", test(nums))
Sample Output:
Original array elements: {0, 15, 16, 17, -14, -13, -12, -11, -10, 18, 19, 20} Largest sum - Positive/Negative numbers of the said array: 105 Original array elements: {0, 3, 4, 5, 9, -22, -44, -11} Largest sum - Positive/Negative numbers of the said array: -77
Explanation:
Here is a breakdown of the above Python code:
- Define Test Function (test function):
- The "test()" function takes a list of numbers as input.
- It initializes variables 'pos_sum' and 'neg_sum' to store the sum of positive and negative numbers, respectively.
- It iterates through each number in the input list, updating the sums accordingly.
- The function returns the largest sum among positive and negative numbers using the max function with key=abs to consider absolute values.
Flowchart:
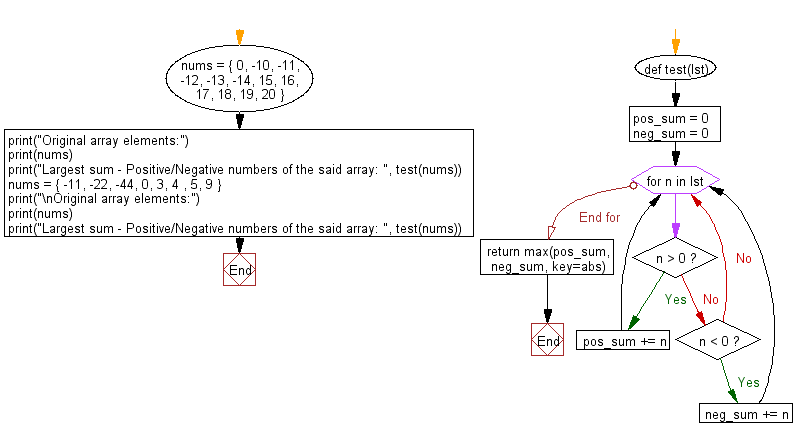
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to find all the factors of a given natural number.
Next: Write a Python program to alternate the case of each letter in a given string and the first letter of the said string must be uppercase.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics