Python: Find all the factors of a given natural number
Python Basic - 1: Exercise-132 with Solution
Write a Python program to find all the factors of a given natural number.
Factors:
The factors of a number are the numbers that divide into it exactly. The number 12 has six factors:
1, 2, 3, 4, 6 and 12
If 12 is divided by any of the six factors then the answer will be a whole number.
For example:
12 ÷ 3 = 4
Sample Solution
Python Code:
#Source https://bit.ly/3w492zp
# Import the 'reduce' function from the 'functools' module.
from functools import reduce
# Define a function 'test' that returns the set of factors for a given number 'n'.
def test(n):
# Use 'reduce' and 'list.__add__' to concatenate lists of factors obtained from the comprehension.
return set(reduce(list.__add__,
([i, n//i] for i in range(1, int(n**0.5) + 1) if n % i == 0)))
'''
Explanation of the test function:
sqrt(x) * sqrt(x) = x. So if the two factors are the same, they're both
the square root. If you make one factor bigger, you have to make the other
factor smaller. This means that one of the two will always be less than or
equal to sqrt(x), so you only have to search up to that point to find one
of the two matching factors. You can then use x / fac1 to get fac2.
The reduce(list.__add__, ...) is taking the little lists of [fac1, fac2]
and joining them together in one long list.
The [i, n/i] for i in range(1, int(sqrt(n)) + 1) if n % i == 0 returns
a pair of factors if the remainder when you divide n by the smaller one
is zero (it doesn't need to check the larger one too; it just gets that
by dividing n by the smaller one.)
The set(...) on the outside is getting rid of duplicates, which only
happens for perfect squares. For n = 4, this will return 2 twice, so
set gets rid of one of them.
'''
# Test cases with different values for the variable 'n'.
n = 1
print("\nOriginal Number:", n)
print("Factors of the said number:", test(n))
n = 12
print("\nOriginal Number:", n)
print("Factors of the said number:", test(n))
n = 100
print("\nOriginal Number:", n)
print("Factors of the said number:", test(n))
Sample Output:
Original Number: 1 Factors of the said number: {1} Original Number: 12 Factors of the said number: {1, 2, 3, 4, 6, 12} Original Number: 100 Factors of the said number: {1, 2, 4, 100, 5, 10, 50, 20, 25}
Explanation:
Here is a breakdown of the above Python code:
- Define Test Function (test function):
- The "test()" function is defined to find the factors of a given number 'n'.
- It uses a list comprehension to generate pairs of factors [i, n//i] for each divisor 'i' in the specified range.
- The 'reduce' function is then used to concatenate these lists into a single list.
- Finally, the 'set' function is applied to eliminate duplicates, as duplicates only occur for perfect squares.
Flowchart:
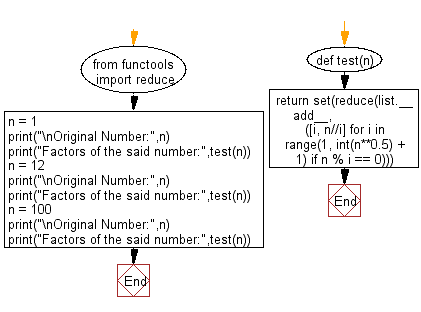
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to count number of zeros and ones in the binary representation of a given integer.
Next: Write a Python program to compute the sum of the negative and positive numbers of an array of integers and display the largest sum.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics