Python: Find the number of combinations
Sum Combination Counter
Write a Python program to find the number of combinations that satisfy p + q + r + s = n where n is a given number <= 4000 and p, q, r, s are between 0 to 1000.
Sample Solution:
Python Code:
# Import the Counter class from the collections module
from collections import Counter
# Print statement to instruct the user to input a positive integer (ctrl+d to exit)
print("Input a positive integer: (ctrl+d to exit)")
# Create a Counter object to store pairs of integers and their counts
pair_dict = Counter()
# Assign counts to pairs of integers based on their minimum and maximum values
for i in range(2001):
pair_dict[i] = min(i, 2000 - i) + 1
# Continuous loop to read input until EOFError (ctrl+d) is encountered
while True:
try:
# Read a positive integer from the user
n = int(input())
# Initialize a variable to store the number of combinations
ans = 0
# Iterate over possible values of 'i' and calculate combinations
for i in range(n + 1):
ans += pair_dict[i] * pair_dict[n - i]
# Print the number of combinations of a, b, c, d
print("Number of combinations of a, b, c, d:", ans)
# Break the loop when EOFError is encountered
except EOFError:
break
Sample Output:
Input a positive integer: (ctrl+d to exit) 252 Number of combinations of a,b,c,d: 2731135
Explanation:
Here is a breakdown of the above Python code:
- Import the Counter class from the "collections" module.
- Print a statement instructing the user to input a positive integer (ctrl+d to exit).
- Create a Counter object (pair_dict) to store pairs of integers and their counts.
- Assign counts to pairs of integers based on their minimum and maximum values.
- Enter a continuous loop to read input until EOFError (ctrl+d) is encountered.
- Read a positive integer from the user.
- Initialize a variable to store the number of combinations (ans).
- Iterate over possible values of 'i' and calculate combinations.
- Print the number of combinations of a, b, c, d.
- Break the loop when EOFError is encountered.
Flowchart:
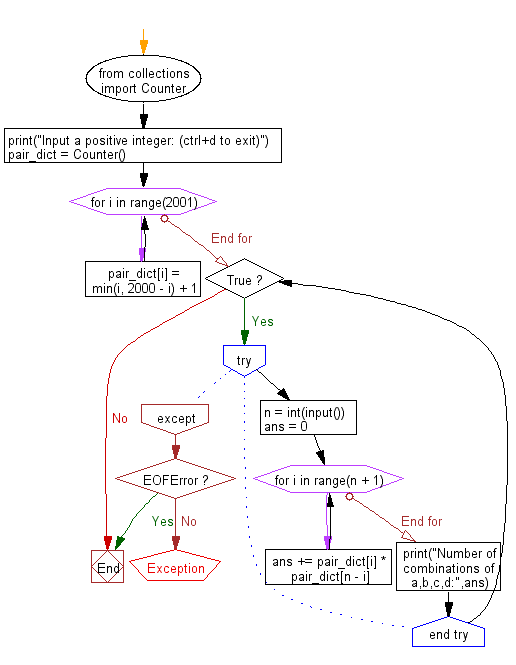
For more Practice: Solve these Related Problems:
- Write a Python program to count the number of quadruple combinations (p, q, r, s) that sum to a given number using nested loops.
- Write a Python program to compute the number of solutions for p + q + r + s = n using a combinatorial approach.
- Write a Python program to count the number of ways to partition an integer n into four non-negative parts using dynamic programming.
- Write a Python program to iterate over a constrained range and count all quadruplets that satisfy the equation p + q + r + s = n.
Go to:
Previous: Write a Python program that compute the maximum value of the sum of the passing integers.
Next: Write a Python program which adds up columns and rows of given table as shown in the specified figure.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.