Python: Compute the maximum value of the sum of the passing integers
Python Basic - 1: Exercise-61 with Solution
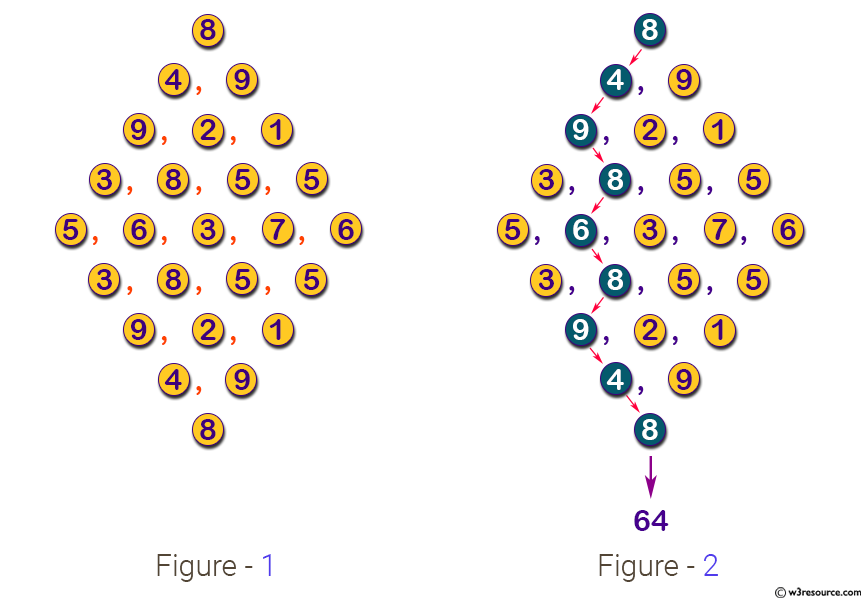
Arrange integers (0 to 99) as narrow hilltop, as illustrated in Figure 1. Reading such data representing huge, when starting from the top and proceeding according to the next rule to the bottom. Write a Python program that compute the maximum value of the sum of the passing integers according to the following rules.
At each step, you can go to the lower left diagonal or the lower right diagonal. For example, in the example of FIG. 1, as shown in FIG. 2, when 8,4,9,8,6,8,9,4,8 is selected and passed, the sum is 64 (8 + 4 + 9) + 8 + 6 + 8 + 9 + 4 + 8 = 64)
Input:
A series of integers separated by commas are given in diamonds. No spaces are included in each line. The input example corresponds to Figure 1. The number of lines of data is less than 100 lines.
Ref: https://bit.ly/3ct00TY
Sample Solution:
Python Code:
# Import the sys module for reading input
import sys
# Print statement to instruct the user to input numbers (ctrl+d to exit)
print("Input the numbers (ctrl+d to exit):")
# Read lines from the standard input and create a list of lists of integers
nums = [list(map(int, l.split(","))) for l in sys.stdin]
# Initialize the maximum value vector with the first row of input
mvv = nums[0]
# Iterate over the remaining rows in a pyramid fashion to find the maximum path sum
for i in range(1, (len(nums) + 1) // 2):
rvv = [0] * (i + 1)
for j in range(i):
rvv[j] = max(rvv[j], mvv[j] + nums[i][j])
rvv[j + 1] = max(rvv[j + 1], mvv[j] + nums[i][j + 1])
mvv = rvv
# Continue iterating over the remaining rows in a reversed pyramid fashion
for i in range((len(nums) + 1) // 2, len(nums)):
rvv = [0] * (len(mvv) - 1)
for j in range(len(rvv)):
rvv[j] = max(mvv[j], mvv[j + 1]) + nums[i][j]
mvv = rvv
# Print the maximum value of the sum of integers passing according to the rule
print("Maximum value of the sum of integers passing according to the rule on one line.")
print(mvv[0])
Sample Output:
Input the numbers (ctrl+d to exit): 8 4, 9 9, 2, 1 3, 8, 5, 5 5, 6, 3, 7, 6 3, 8, 5, 5 9, 2, 1 4, 9 8 Maximum value of the sum of integers passing according to the rule on one line. 64
Explanation:
Here is a breakdown of the above Python code:
- Import the "sys" module for reading input.
- Print a statement instructing the user to input numbers (ctrl+d to exit).
- Read lines from the standard input and create a list of lists of integers (nums).
- Initialize the maximum value vector (mvv) with the first row of input.
- Iterate over the remaining rows in a pyramid fashion to find the maximum path sum.
- Continue iterating over the remaining rows in a reversed pyramid fashion.
- Print the maximum value of the sum of integers passed according to the rule.
Flowchart:
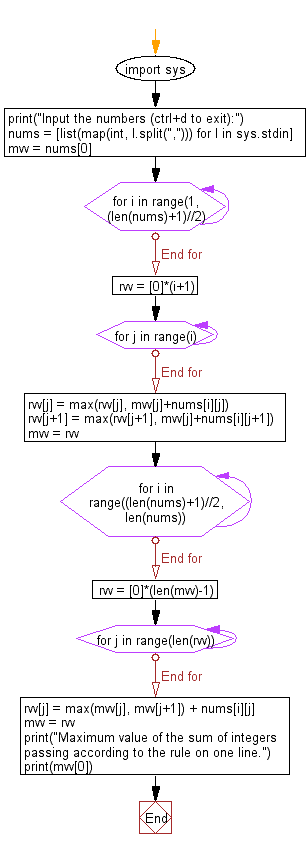
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to cut out words of 3 to 6 characters length from a given sentence not more than 1024 characters.
Next: Write a Python program to find the number of combinations that satisfy p + q + r + s = n where n is a given number <= 4000 and p, q, r, s in the range of 0 to 1000.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics