Python: Find and print the first 10 happy numbers
Python Basic - 1: Exercise-67 with Solution
First 10 Happy Numbers
From Wikipedia,
A happy number is defined by the following process:
Starting with any positive integer, replace the number by the sum of the squares of its digits, and repeat the process until the number equals 1 (where it will stay), or it loops endlessly in a cycle which does not include 1. Those numbers for which this process ends in 1 are happy numbers, while those that do not end in 1 are unhappy numbers.
Write a Python program to find and print the first 10 happy numbers.
Sample Solution:
Python Code:
# Function to check if a number is a Happy Number
def happy_numbers(n):
past = set() # Set to store previously encountered numbers during the process
while n != 1: # Continue the process until the number becomes 1 (a Happy Number) or a cycle is detected
n = sum(int(i) ** 2 for i in str(n)) # Calculate the sum of squares of each digit in the number
if n in past: # If the current number has been encountered before, it forms a cycle
return False # The number is not a Happy Number
past.add(n) # Add the current number to the set of past numbers
return True # If the process reaches 1, the number is a Happy Number
# Print the first 10 Happy Numbers less than 500
print([x for x in range(500) if happy_numbers(x)][:10])
Sample Output:
[1, 7, 10, 13, 19, 23, 28, 31, 32, 44]
Explanation:
Here is a breakdown of the above Python code:
- Define a function named "happy_numbers()" that takes a number 'n' as input.
- Initialize a set called 'past' to keep track of previously encountered numbers during the process.
- Use a while loop to continue the process until the number becomes 1 (a Happy Number) or a cycle is detected.
- Inside the loop, calculate the sum of the squares of each digit in the current number.
- Check if the current number has been encountered before. If yes, it forms a cycle, and the number is not a Happy Number.
- Add the current number to the set of past numbers.
- Return 'True' if the process reaches 1, indicating that the number is a Happy Number.
- Use a list comprehension to generate the first 10 Happy Numbers less than 500 and print the result.
Visual Presentation:
Flowchart:
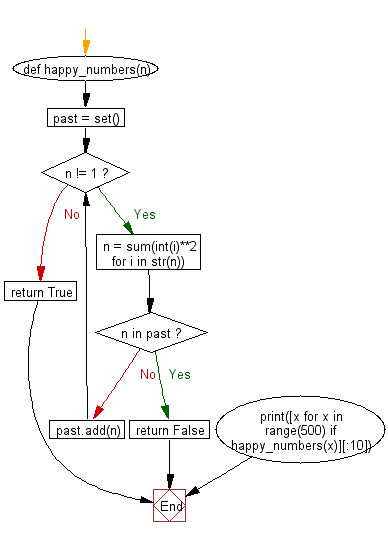
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Write a Python program to check whether a number is "happy" or not.
Next: Write a Python program to count the number of prime numbers less than a given non-negative number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/basic/python-basic-1-exercise-67.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics