Python: Check whether a given employee code is exactly 8 digits or 12 digits
Employee Code Validator
Write a Python program to check whether a given employee code is exactly 8 digits or 12 digits. Return True if the employee code is valid and False if it's not.
Sample Solution:
Python Code:
# Define a function named is_valid_emp_code that takes an employee code (emp_code) as an argument.
def is_valid_emp_code(emp_code):
# Check if the length of the employee code is either 8 or 12 and if it consists only of digits.
return len(emp_code) in [8, 12] and emp_code.isdigit()
# Test the function with different employee codes and print the results.
print(is_valid_emp_code('12345678'))
print(is_valid_emp_code('1234567j'))
print(is_valid_emp_code('12345678j'))
print(is_valid_emp_code('123456789123'))
print(is_valid_emp_code('123456abcdef'))
Sample Output:
True False False True False
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named is_valid_emp_code that takes an employee code (emp_code) as an argument.
- Return Statement:
- The function returns 'True' if the length of the employee code is either 8 or 12 and if the code consists only of digits. Otherwise, it returns 'False'.
- Test cases:
- The function is tested with different employee codes using print(is_valid_emp_code(...)).
Pictorial Presentation:
Flowchart:
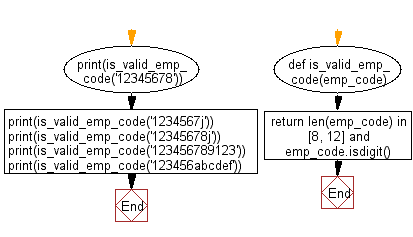
For more Practice: Solve these Related Problems:
- Write a Python program to validate an employee code ensuring it consists only of digits and is exactly 8 or 12 characters long.
- Write a Python program to check if a given employee code is valid based on its length and numeric composition.
- Write a Python program to verify that an input string is a valid employee code by matching it against allowed lengths using regular expressions.
- Write a Python program to determine if an employee code meets the criteria of being numeric and either 8 or 12 digits long.
Go to:
Previous: Write a Python program to count the number of equal numbers from three given integers.
Next: Write a Python program that accept two strings and test if the letters in the second string are present in the first string.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.