Python Bisect: Locate the left insertion point for a specified value in sorted order
1. Left Insertion Point
Write a Python program to locate the left insertion point for a specified value in sorted order.
Sample Solution:
Python Code:
import bisect
def index(a, x):
i = bisect.bisect_left(a, x)
return i
a = [1,2,4,5]
print(index(a, 6))
print(index(a, 3))
Sample Output:
4 2
Flowchart:
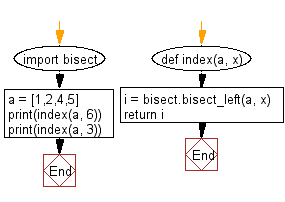
For more Practice: Solve these Related Problems:
- Write a Python program that uses bisect_left to determine the insertion index for a target in a sorted list and then inserts the target at that index.
- Write a Python script to implement your own bisect_left function recursively and test it against Python’s bisect_left for various target values.
- Write a Python program to find the left insertion point for a target in a sorted list of floating-point numbers with duplicate entries and print the index.
- Write a Python function that, given a sorted list and a target, returns the leftmost index where the target can be inserted without breaking the order, and compare its output with the built-in bisect_left.
Go to:
Previous: Python Bisect Home.
Next: Write a Python program to locate the right insertion point for a specified value in sorted order.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.