Python Bisect: Locate the right insertion point for a specified value in sorted order
2. Right Insertion Point
Write a Python program to locate the right insertion point for a specified value in sorted order.
Sample Solution:
Python Code:
import bisect
def index(a, x):
i = bisect.bisect_right(a, x)
return i
a = [1,2,4,7]
print(index(a, 6))
print(index(a, 3))
Sample Output:
3 2
Flowchart:
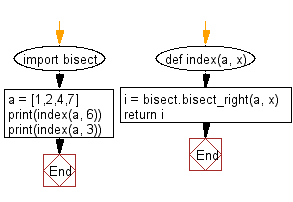
For more Practice: Solve these Related Problems:
- Write a Python program to use bisect_right to find the insertion point for a target value in a sorted list and then insert the target at that position.
- Write a Python script to implement a custom bisect_right function iteratively and compare its result with Python’s bisect_right on various test cases.
- Write a Python program to determine the rightmost insertion index for a given target in a sorted list containing duplicate values using bisect_right.
- Write a Python function that returns the right insertion point for a target in a sorted list and demonstrates the difference between bisect_left and bisect_right.
Go to:
Previous: Write a Python program to locate the left insertion point for a specified value in sorted order.
Next: Write a Python program to insert items into a list in sorted order.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.