Python: Test the third Tuesday of a month
20. Third Tuesday Tester
Write a Python program to test the third Tuesday of a month.
Sample Solution:
Python Code:
# Import the datetime class from the datetime module
from datetime import datetime
# Define a function named is_third_tuesday that takes a date string (s) as input
def is_third_tuesday(s):
# Convert the input date string to a datetime object using the specified format '%b %d, %Y'
d = datetime.strptime(s, '%b %d, %Y')
# Check if the weekday of the date is Tuesday (1) and the day of the month is between 15 and 21
# This condition ensures that the date is the third Tuesday of the month
return d.weekday() == 1 and 14 < d.day < 22
# Print the result of calling is_third_tuesday with different date strings
print(is_third_tuesday('Jun 23, 2015')) # False
print(is_third_tuesday('Jun 16, 2015')) # True
print(is_third_tuesday('Jul 21, 2015')) # False
Output:
False True True
Explanation:
In the exercise above,
- The code imports the "datetime" class from the "datetime" module.
- Define the function to check for the third Tuesday:
- It defines a function named "is_third_tuesday()" that takes a date string (s) as input.
- Within this function,
- It converts the input date string to a "datetime" object using the "strptime()" method, specifying the date format as '%b %d, %Y'.
- It checks if the weekday of the date is Tuesday (1) and if the day of the month is between 15 and 21. This condition ensures that the date is the third Tuesday of the month.
- Finally, it prints the result of calling "is_third_tuesday()" function with different date strings.
- For each date string, it prints 'True' if the date is the third Tuesday of the month and 'False' otherwise.
Flowchart:
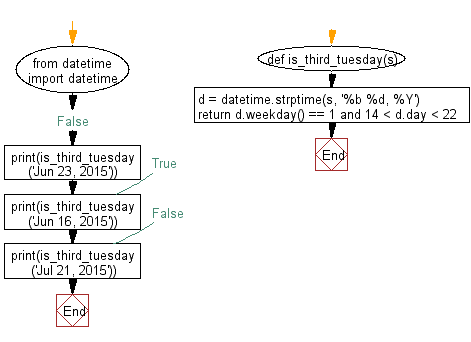
For more Practice: Solve these Related Problems:
- Write a Python program to verify whether the third Tuesday of a given month falls on the 15th day.
- Write a Python function that checks if a specified date is the third Tuesday of its month and returns a boolean value.
- Write a Python script to calculate the date of the third Tuesday for a given month and then compare it with a provided date.
- Write a Python program to determine the third Tuesday of the month and then output the week number of that Tuesday.
Go to:
.Previous: Write a Python program to get the date of the last Tuesday.
Next: Write a Python program to get the last day of a specified year and month.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.