Python: Find out what the epoch is on a given platform
55. Epoch Info and Conversion
The epoch is the point where time starts, and is platform dependent. For Unix, the epoch is January 1, 1970, 00:00:00 (UTC). Write a Python program to find out what the epoch is on a given platform. Convert a given time in seconds since the epoch.
Sample Solution:
Python Code:
# Import the time module
import time
# Print a message indicating the epoch on a given platform
print("\nEpoch on a given platform:")
# Print the time structure representing the epoch (January 1, 1970, 00:00:00 UTC)
print(time.gmtime(0))
# Print a message indicating time in seconds since the epoch
print("\nTime in seconds since the epoch:")
# Print the time structure representing 36000 seconds after the epoch
print(time.gmtime(36000))
Output:
Epoch on a given platform: time.struct_time(tm_year=1970, tm_mon=1, tm_mday=1, tm_hour=0, tm_min=0, tm_sec=0, tm_wday=3, tm_yday=1, tm_isdst=0) Time in seconds since the epoch: time.struct_time(tm_year=1970, tm_mon=1, tm_mday=1, tm_hour=10, tm_min=0, tm_sec=0, tm_wday=3, tm_yday=1, tm_isdst=0)
Explanation:
In the exercise above,
- The code imports the "time" module
- It prints a message indicating the epoch on a given platform.
- It uses the "time.gmtime()" function to convert the time in seconds since the epoch to a time structure representing UTC time.
- In the first "print" statement, "time.gmtime(0)" represents the time structure for the epoch, i.e., January 1, 1970, 00:00:00 UTC.
- It prints a message indicating the time in seconds since the epoch.
- It again uses the "time.gmtime()" function to convert the time in seconds (36000 seconds) since the epoch to a time structure representing UTC time.
- In the second "print" statement, "time.gmtime(36000)" represents the time structure for 36000 seconds after the epoch.
Flowchart:
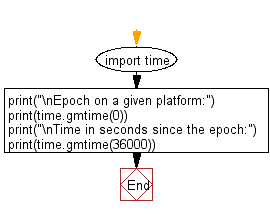
For more Practice: Solve these Related Problems:
- Write a Python program to display the Unix epoch start time and then convert a given number of seconds since the epoch to a readable date.
- Write a Python script to compute the current time in seconds since the epoch and then convert it back to a local datetime.
- Write a Python function to take an input of seconds since the epoch, convert it to a datetime object, and then format the result as a string.
- Write a Python program to find the epoch time on your system and then calculate the elapsed time from the epoch to a given date.
Go to:
Previous: Write a Python program to set the default timezone used by all date/time functions.
Next: Write a Python program to get time values with components using local time and gmtime
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.