Python: Set the default timezone used by all date/time functions
54. Set Default Timezone
Write a Python program to set the default timezone used by all date/time functions.
Sample Solution:
Python Code:
# Import the os and time modules
import os, time
# Print the current time in the default timezone before the timezone change
print(time.strftime('%Y-%m-%d %H:%M:%S')) # before timezone change
# Set the new timezone to 'America/Los_Angeles'
os.environ['TZ'] = 'America/Los_Angeles'
# Apply the timezone change
time.tzset()
# Print the current time in the new timezone after the timezone change
print(time.strftime('%Y-%m-%d %H:%M:%S')) # after timezone change
Output:
2020-07-21 13:09:47 2020-07-21 06:09:47
Explanation:
In the exercise above,
- The code imports the "os" and "time" modules. time.
- It prints the current time in the default timezone using "time.strftime('%Y-%m-%d %H:%M:%S')". This function formats the time according to the given format string, which represents the year, month, day, hour, minute, and second.
- It sets the new timezone to 'America/Los_Angeles' by updating the 'TZ' environment variable in the "os.environ" dictionary.
- It applies the timezone change using "time.tzset()", which refreshes the timezone settings.
- It prints the current time in the new timezone after the timezone change using the same "time.strftime('%Y-%m-%d %H:%M:%S')" function.
Flowchart:
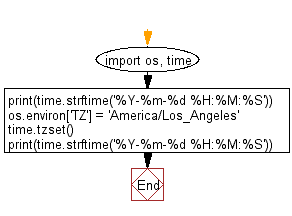
For more Practice: Solve these Related Problems:
- Write a Python program to set the default timezone for all datetime operations to a specified timezone and then display the current time in that timezone.
- Write a Python script to configure the default timezone in your application and then convert a local datetime to that timezone.
- Write a Python function to change the system’s default timezone temporarily and then print the current time in the new timezone.
- Write a Python program to set and reset the default timezone using the pytz module and then demonstrate the effect on datetime outputs.
Go to:
Previous: Write a Python program to get the first and last second.
Next: The epoch is the point where the time starts, and is platform dependent. For Unix, the epoch is January 1, 1970, 00:00:00 (UTC). Write a Python program to find out what the epoch is on a given platform. Also convert a given time in seconds since the epoch.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.