Python: Validate a Gregorian date
53. Gregorian Date Validator
Write a Python program to validate a Gregorian date. The month is between 1 and 12 inclusive, the day is within the allowed number of days for the given month. Leap year’s are taken into consideration. The year is between 1 and 32767 inclusive.
Sample Solution:
Python Code:
# Import the datetime module
import datetime
# Define a function named check_date which checks if the provided date is valid
def check_date(m, d, y):
try:
# Convert month, day, and year to integers
m, d, y = map(int, (m, d, y))
# Attempt to create a datetime object with the provided date components
datetime.date(y, m, d)
# If successful, return True (valid date)
return True
except ValueError:
# If ValueError is raised (invalid date), return False
return False
# Test the check_date function with different inputs
print(check_date(11, 11, 2002)) # Valid date: November 11, 2002
print(check_date('11', '11', '2002')) # Valid date: November 11, 2002 (provided as strings)
print(check_date(13, 11, 2002)) # Invalid date: February 13, 2002 (day exceeds maximum for February)
Output:
True True False
Explanation:
In the exercise above,
- The code imports the "datetime" module.
- It defines a function named "check_date()" which takes three parameters representing the month, day, and year respectively.
- Inside the function:
- It attempts to convert the month, day, and year to integers using the "map()" function and 'int' constructor.
- It attempts to create a datetime.date object with the provided date components.
- If successful (no "ValueError" is raised), it returns 'True' indicating a valid date.
- If a "ValueError" is raised (invalid date), it catches the exception and returns 'False'.
- It tests the "check_date()" function with different inputs:
- check_date(11, 11, 2002) checks for November 11, 2002, which is a valid date.
- check_date('11', '11', '2002') checks for the same date, but with the components provided as strings.
- check_date(13, 11, 2002) checks for February 13, 2002, which is an invalid date because the day exceeds the maximum for February.
Flowchart:
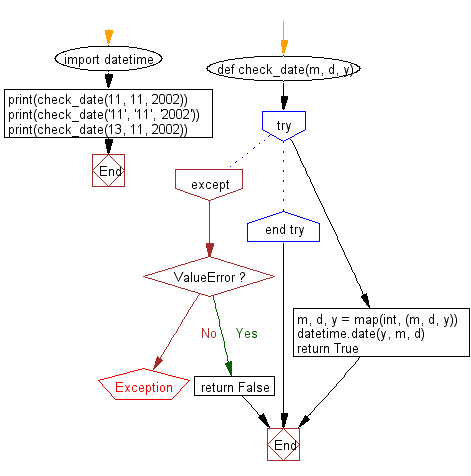
For more Practice: Solve these Related Problems:
- Write a Python program to validate if an input date is a valid Gregorian date, considering leap years and month-day limits.
- Write a Python script to check whether a given date string represents a valid Gregorian date and then return a boolean result.
- Write a Python function to validate a date by ensuring the month is 1–12 and the day is valid for that month, including leap year adjustments.
- Write a Python program to prompt the user for a date and then verify its validity as a Gregorian date using custom logic.
Go to:
Previous: Write a Python program to get the first and last second.
Next: Write a Python program to set the default timezone used by all date/time functions.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.