Python: Remove a specified dictionary from a given list
48. Remove Specified Dictionary from a List
Write a Python program to remove a specified dictionary from a given list.
Visual Presentation:
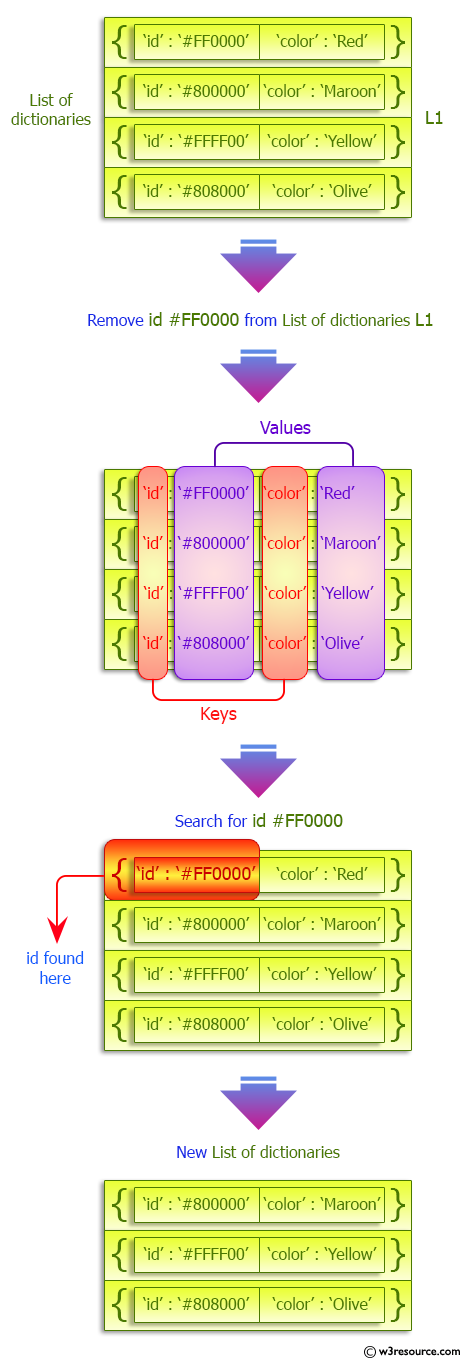
Sample Solution:
Python Code:
# Define a function 'remove_dictionary' that removes a dictionary with a specific 'id' from a list of dictionaries.
def remove_dictionary(colors, r_id):
# Use a list comprehension to filter out dictionaries with an 'id' not equal to 'r_id'.
# The filtered result is assigned back to the 'colors' list, effectively removing the dictionary with the specified 'id'.
colors[:] = [d for d in colors if d.get('id') != r_id]
return colors
# Create a list of dictionaries 'colors' with 'id' and 'color' as keys.
colors = [
{"id": "#FF0000", "color": "Red"},
{"id": "#800000", "color": "Maroon"},
{"id": "#FFFF00", "color": "Yellow"},
{"id": "#808000", "color": "Olive"}
]
# Print a message indicating the start of the code section and the original list of dictionaries.
print('Original list of dictionary:')
print(colors)
# Define the 'r_id' variable with the value of the 'id' to be removed from the list.
r_id = "#FF0000"
# Print a message indicating the intention to remove the dictionary with 'r_id' from the list.
print("\nRemove id", r_id, "from the said list of dictionary:")
# Call the 'remove_dictionary' function to remove the dictionary with the specified 'id'.
# Print the resulting list of dictionaries after the removal.
print(remove_dictionary(colors, r_id))
Sample Output:
Original list of dictionary: [{'id': '#FF0000', 'color': 'Red'}, {'id': '#800000', 'color': 'Maroon'}, {'id': '#FFFF00', 'color': 'Yellow'}, {'id': '#808000', 'color': 'Olive'}] Remove id #FF0000 from the said list of dictionary: [{'id': '#800000', 'color': 'Maroon'}, {'id': '#FFFF00', 'color': 'Yellow'}, {'id': '#808000', 'color': 'Olive'}]
Flowchart:
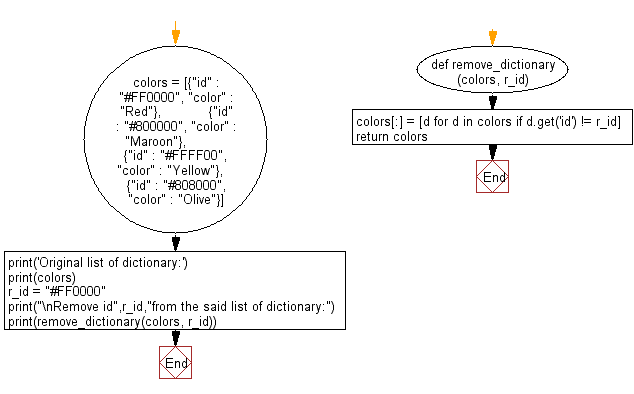
For more Practice: Solve these Related Problems:
- Write a Python program to filter out a dictionary from a list based on a matching key-value pair using list comprehension.
- Write a Python program to remove a dictionary with a specified key from a list of dictionaries by iterating over the list.
- Write a Python program to implement a function that deletes dictionaries from a list that match given criteria.
- Write a Python program to use filter() to exclude dictionaries that contain a specific key-value combination.
Go to:
Previous: Write a Python program to split a given dictionary of lists into list of dictionaries.
Next: Write a Python program to convert string values of a given dictionary, into integer/float datatypes.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.