Python: Convert string values of a given dictionary, into integer/float datatypes
49. Convert String Values in Dictionary to Numeric Types
Write a Python program to convert string values of a given dictionary into integer/float datatypes.
Visual Presentation:
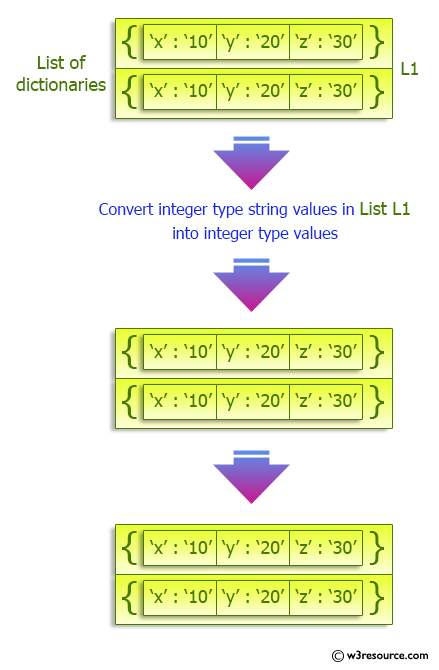
Sample Solution:
Python Code:
# Define a function 'convert_to_int' that converts string values in a list of dictionaries to integers.
def convert_to_int(lst):
# Use a list comprehension to iterate through each dictionary 'b' in the list 'lst'.
# For each dictionary, convert the string values to integers and create a new dictionary.
result = [dict([a, int(x)] for a, x in b.items()) for b in lst]
return result
# Define a function 'convert_to_float' that converts string values in a list of dictionaries to floats.
def convert_to_float(lst):
# Use a list comprehension to iterate through each dictionary 'b' in the list 'lst'.
# For each dictionary, convert the string values to floats and create a new dictionary.
result = [dict([a, float(x)] for a, x in b.items()) for b in lst]
return result
# Create a list of dictionaries 'nums' with keys and string values.
nums_int = [
{'x': '10', 'y': '20', 'z': '30'},
{'p': '40', 'q': '50', 'r': '60'}
]
# Print a message indicating the start of the code section and the original list of dictionaries.
print("Original list:")
print(nums_int)
# Print a message indicating the intention to convert string values to integers.
print("\nString values of a given dictionary, into integer types:")
# Call the 'convert_to_int' function to convert string values to integers and print the resulting list of dictionaries.
print(convert_to_int(nums_int))
# Create a list of dictionaries 'nums' with keys and string values in floating-point format.
nums_float = [
{'x': '10.12', 'y': '20.23', 'z': '30'},
{'p': '40.00', 'q': '50.19', 'r': '60.99'}
]
# Print a message indicating the start of the code section and the original list of dictionaries.
print("\nOriginal list:")
print(nums_float)
# Print a message indicating the intention to convert string values to floats.
print("\nString values of a given dictionary, into float types:")
# Call the 'convert_to_float' function to convert string values to floats and print the resulting list of dictionaries.
print(convert_to_float(nums_float))
Sample Output:
Original list: [{'x': '10', 'y': '20', 'z': '30'}, {'p': '40', 'q': '50', 'r': '60'}] String values of a given dictionary, into integer types: [{'x': 10, 'y': 20, 'z': 30}, {'p': 40, 'q': 50, 'r': 60}] Original list: [{'x': '10.12', 'y': '20.23', 'z': '30'}, {'p': '40.00', 'q': '50.19', 'r': '60.99'}] String values of a given dictionary, into float types: [{'x': 10.12, 'y': 20.23, 'z': 30.0}, {'p': 40.0, 'q': 50.19, 'r': 60.99}]
Flowchart:
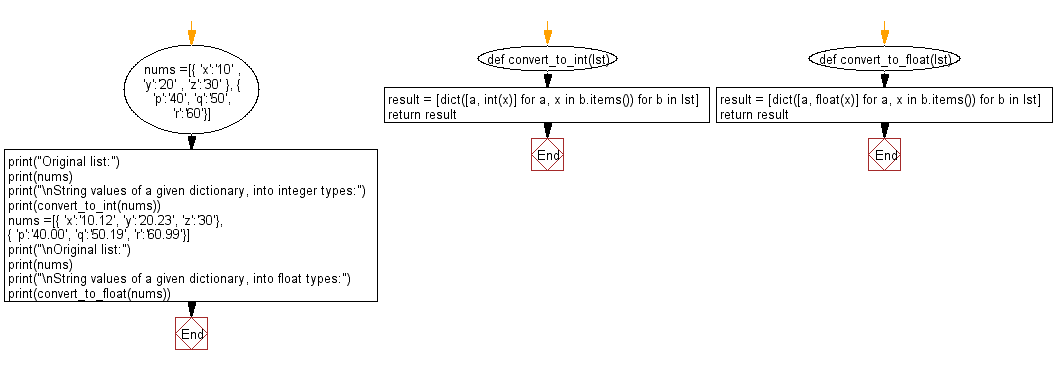
For more Practice: Solve these Related Problems:
- Write a Python program to iterate over a dictionary and convert numeric string values to integers using int().
- Write a Python program to update dictionary values to float if they contain a decimal point, otherwise to int.
- Write a Python program to use try/except to safely cast dictionary string values to numeric types.
- Write a Python function that checks each value of a dictionary and converts it to a numeric type if possible.
Go to:
Previous: Write a Python program to remove a specified dictionary from a given list.
Next: A Python Dictionary contains List as value. Write a Python program to clear the list values in the said dictionary.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.