Python: Creates a dictionary with the same keys as the given dictionary and values generated by running the given function for each value
74. Create Dictionary with Same Keys and Function-Transformed Values
Write a Python program to create a dictionary with the same keys as the given dictionary and values generated by running the given function for each value.
Sample Solution:
Python Code:
# Define a function 'test' that takes a dictionary 'obj' and a function 'fn'.
def test(obj, fn):
# Use a dictionary comprehension to apply the function 'fn' to each value in the 'obj' dictionary.
# The result is a new dictionary with the same keys, where each value is transformed by 'fn'.
return dict((k, fn(v)) for k, v in obj.items())
# Create a dictionary 'users' where each key corresponds to a user and has associated data as a dictionary.
users = {
'Theodore': {'user': 'Theodore', 'age': 45},
'Roxanne': {'user': 'Roxanne', 'age': 15},
'Mathew': {'user': 'Mathew', 'age': 21},
}
# Print the original dictionary elements.
print("\nOriginal dictionary elements:")
print(users)
# Call the 'test' function to create a new dictionary with the same keys, but with 'age' values extracted.
print("\nDictionary with the same keys:")
print(test(users, lambda u: u['age']))
Sample Output:
Original dictionary elements: {'Theodore': {'user': 'Theodore', 'age': 45}, 'Roxanne': {'user': 'Roxanne', 'age': 15}, 'Mathew': {'user': 'Mathew', 'age': 21}} Dictionary with the same keys: {'Theodore': 45, 'Roxanne': 15, 'Mathew': 21}
Flowchart:
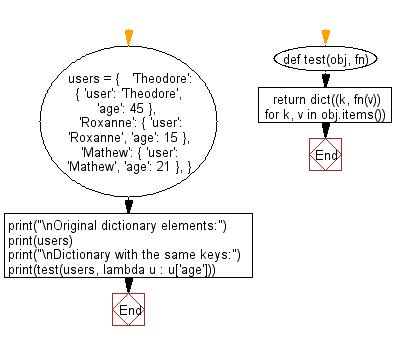
For more Practice: Solve these Related Problems:
- Write a Python program to apply a given function to each value of a dictionary and return a new dictionary with the same keys and transformed values.
- Write a Python program to use dictionary comprehension to map over a dictionary's values with a provided function.
- Write a Python program to implement a function that takes a dictionary and a transformation function, returning a new dictionary with updated values.
- Write a Python program to combine lambda functions with dictionary comprehension to modify all values in a dictionary.
Go to:
Previous: Write a Python program to convert a list of dictionaries into a list of values corresponding to the specified key.
Next: Write a Python program to find all keys in the provided dictionary that have the given value.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.