Python: Find all keys in the provided dictionary that have the given value
75. Find All Keys with a Given Value
Write a Python program to find all keys in a dictionary that have the given value.
Sample Solution:
Python Code:
# Define a function 'test' that takes a dictionary 'dict' and a value 'val'.
def test(dict, val):
# Use a list comprehension to find all keys in the dictionary where the corresponding value is equal to 'val'.
return list(key for key, value in dict.items() if value == val)
# Create a dictionary 'students' where each key corresponds to a student's name, and the associated value is their age.
students = {
'Theodore': 19,
'Roxanne': 20,
'Mathew': 21,
'Betty': 20
}
# Print the original dictionary elements.
print("\nOriginal dictionary elements:")
print(students)
# Call the 'test' function to find all keys in the dictionary that have the specified value, which is 20 in this case.
print("\nFind all keys in the said dictionary that have the specified value:")
print(test(students, 20))
Sample Output:
Original dictionary elements: {'Theodore': 19, 'Roxanne': 20, 'Mathew': 21, 'Betty': 20} Find all keys in the said dictionary that have the specified value: ['Roxanne', 'Betty']
Flowchart:
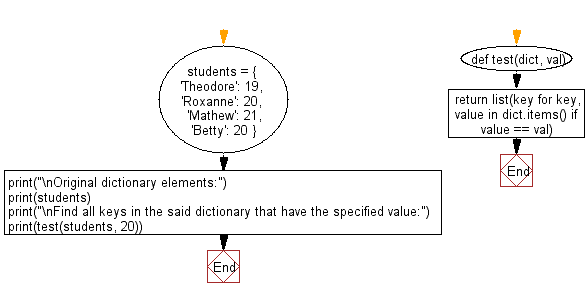
For more Practice: Solve these Related Problems:
- Write a Python program to iterate over a dictionary and collect all keys whose value matches a given target.
- Write a Python program to use list comprehension to extract keys with a specified value from a dictionary.
- Write a Python program to implement a function that returns a list of keys with a particular value from a dictionary.
- Write a Python program to compare each key-value pair and output keys if the value equals a specified number.
Go to:
Previous: Write a Python program to create a dictionary with the same keys as the given dictionary and values generated by running the given function for each value.
Next: Write a Python program to combine two lists into a dictionary, where the elements of the first one serve as the keys and the elements of the second one serve as the values. The values of the first list need to be unique and hashable.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.