Python: Combine two lists into a dictionary, where the elements of the first one serve as the keys and the elements of the second one serve as the values
Python dictionary: Exercise-76 with Solution
Write a Python program to combine two lists into a dictionary. The elements of the first one serve as keys and the elements of the second one serve as values. Each item in the first list must be unique and hashable.
- Use zip() in combination with dict() to combine the values of the two lists into a dictionary.
Sample Solution:
Python Code:
# Define a function 'test' that takes two lists, 'keys' and 'values', and combines them into a dictionary.
def test(keys, values):
# Use the 'zip' function to pair elements from 'keys' and 'values' lists, and then convert the result into a dictionary.
return dict(zip(keys, values))
# Create two lists, 'l1' and 'l2', containing keys and values, respectively.
l1 = ['a', 'b', 'c', 'd', 'e', 'f']
l2 = [1, 2, 3, 4, 5]
# Print the original lists.
print("Original lists:")
print(l1)
print(l2)
# Call the 'test' function to combine the values of the two lists into a dictionary.
print("\nCombine the values of the said two lists into a dictionary:")
print(test(l1, l2))
Sample Output:
Original lists: ['a', 'b', 'c', 'd', 'e', 'f'] [1, 2, 3, 4, 5] Combine the values of the said two lists into a dictionary: {'a': 1, 'b': 2, 'c': 3, 'd': 4, 'e': 5}
Flowchart:
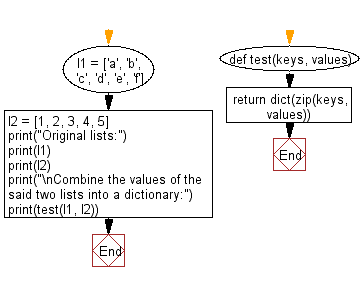
Python Code Editor:
Previous: Write a Python program to find all keys in the provided dictionary that have the given value.
Next: Write a Python program to convert given a dictionary to a list of tuples.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/dictionary/python-data-type-dictionary-exercise-76.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics