Python Function: Reverse a given bytes object
Python Bytes and Byte Arrays Data Type: Exercise-6 with Solution
Write a Python function to reverse a given bytes object.
Sample Solution:
Code:
def reverse_bytes(byte_obj):
reversed_bytes = byte_obj[::-1]
return reversed_bytes
def main():
try:
original_bytes = b"Python Exercises!"
reversed_result = reverse_bytes(original_bytes)
print("Original Bytes:", original_bytes)
print("Reversed Bytes:", reversed_result)
print("Reversed String:", reversed_result.decode("utf-8"))
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Original Bytes: b'Python Exercises!' Reversed Bytes: b'!sesicrexE nohtyP' Reversed String: !sesicrexE nohtyP
In the exercise above, the "reverse_bytes()" function takes a bytes object as input and reverses it using slicing with a step of -1. In the "main()" function, a sample bytes object is provided and the reversed bytes and the reversed string are printed.
Flowchart:
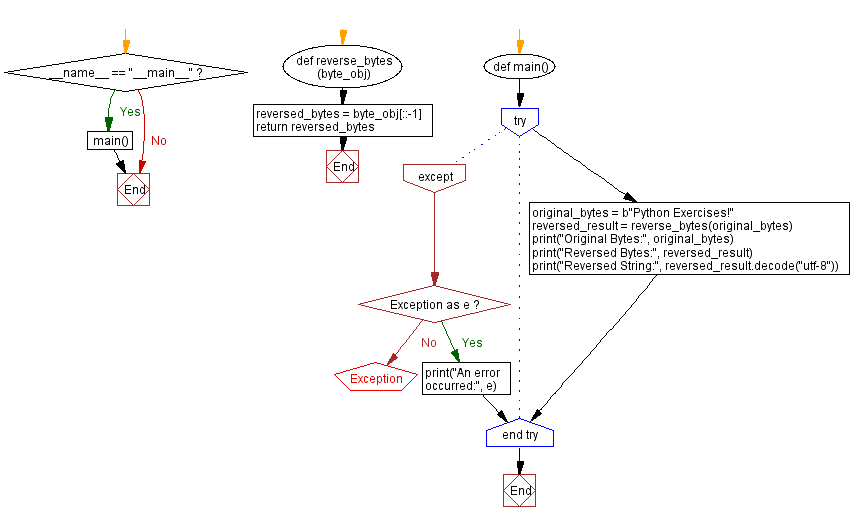
Previous: Convert hexadecimal string to bytes.
Next: Python Program: Check equality of bytes objects.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics