Python Program: Convert hexadecimal string to bytes
5. Hexadecimal to Bytes Conversion
Write a Python program that converts a hexadecimal string into a bytes sequence.
Sample Solution:
Code:
def hex_str_to_bytes(hex_string):
try:
bytes_sequence = bytes.fromhex(hex_string)
return bytes_sequence
except ValueError as ve:
print("Error:", ve)
return None
def main():
try:
hex_string = "4861636b657220436f6465"
bytes_result = hex_str_to_bytes(hex_string)
if bytes_result is not None:
print("Hexadecimal String:", hex_string)
print("Bytes Sequence:", bytes_result)
print("Decoded String:", bytes_result.decode("utf-8"))
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Hexadecimal String: 4861636b657220436f6465 Bytes Sequence: b'Hacker Code' Decoded String: Hacker Code
In the exercise above, the "hex_string_to_bytes()" function takes a hexadecimal string as input and converts it into a bytes sequence using the "bytes.fromhex()" method. In the "main()" function, a sample hexadecimal string is provided and the resultant bytes sequence and decoded string are printed.
Flowchart:
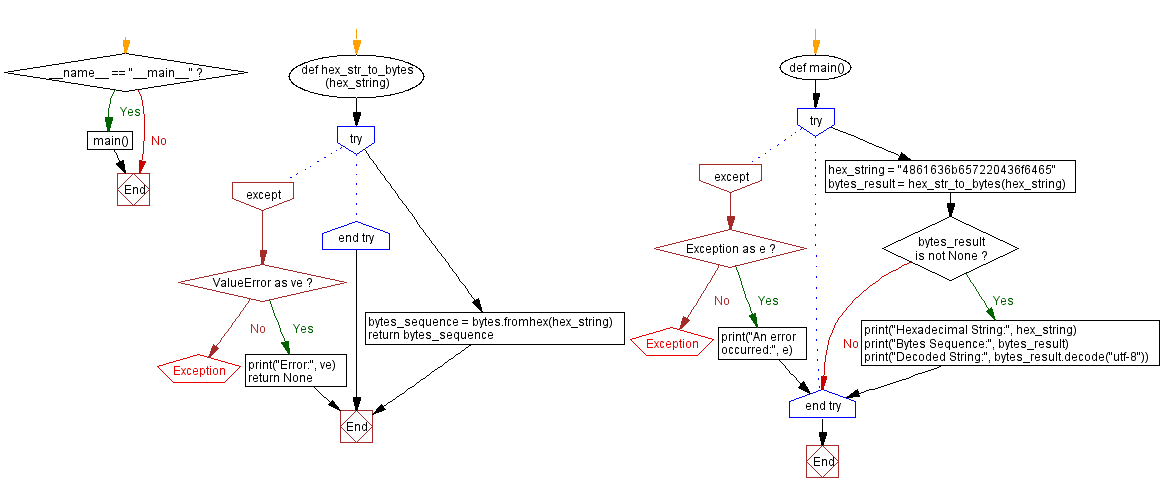
For more Practice: Solve these Related Problems:
- Write a Python program to convert a hexadecimal string into a bytes sequence and then display the bytes as a list of integers.
- Write a Python script to take a hex string input, convert it to bytes, and then encode the bytes back into a hex string to verify the conversion.
- Write a Python function that converts a hexadecimal string to a bytes object and then decodes it using UTF-8 if possible.
- Write a Python program to process a hexadecimal string with mixed uppercase and lowercase letters and convert it into a standardized bytes object.
Go to:
Previous: Read and modify image as bytes.
Next: Python Function: Reverse a given bytes object.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.