Python Program: Read and modify image as bytes
4. Image File Bytes Manipulation
Write a Python program that reads an image file into a bytes object and saves a modified copy.
Sample Solution:
Code:
def read_image(file_path):
with open(file_path, "rb") as file:
image_bytes = file.read()
return image_bytes
def save_modified_image(file_path, modified_bytes):
with open(file_path, "wb") as file:
file.write(modified_bytes)
def main():
try:
input_image_path = "flowchart_image.png"
output_image_path = "output_image.png"
# Read the image into bytes
original_image_bytes = read_image(input_image_path)
# Modify the bytes (e.g., adding watermark)
modified_image_bytes = original_image_bytes + b"Watermark"
# Save the modified image
save_modified_image(output_image_path, modified_image_bytes)
print("Image read and modified successfully.")
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Image read and modified successfully.
In the exercise above the "read_image()" function reads an image file into bytes using the "rb" (read binary) mode. The "save_modified_image()" function saves a modified bytes object into an image file using the "wb" (write binary) mode. The "main()" function demonstrates the usage of these functions by reading an input image, changing the bytes (in this case, adding a watermark), and saving the modified image.
Flowchart:
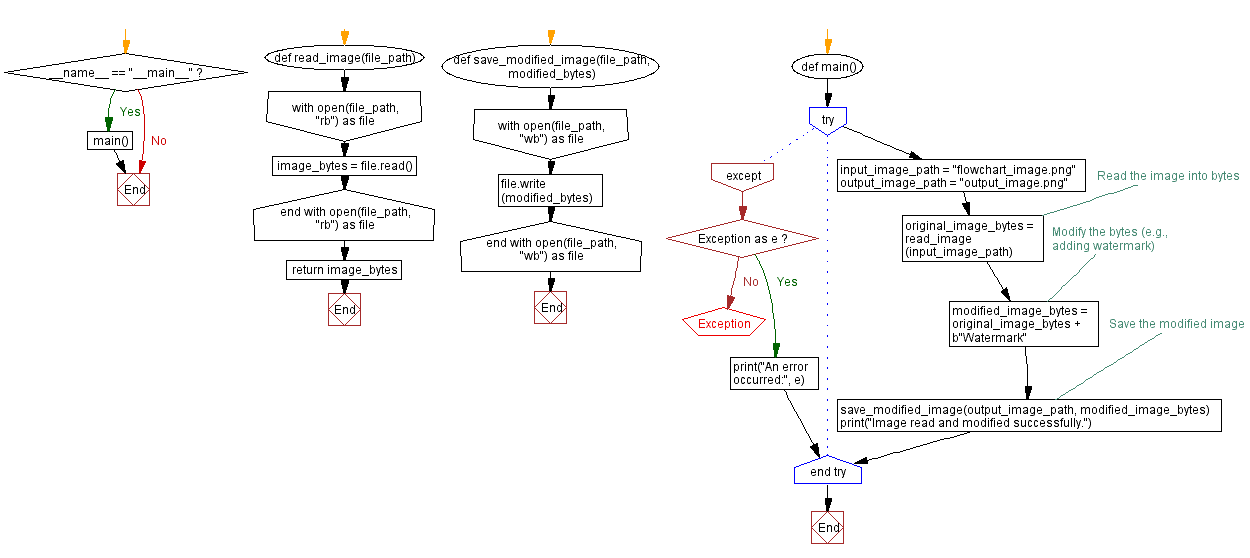
For more Practice: Solve these Related Problems:
- Write a Python program to read an image file into a bytes object, modify some of its bytes, and then save the result as a new image file.
- Write a Python script to open an image file in binary mode, convert its bytes to a base64 string, and then decode it back to image bytes.
- Write a Python program to read an image as bytes, apply a simple transformation (e.g., bitwise NOT), and save the transformed image.
- Write a Python function to read an image file into bytes, simulate a corruption by altering specific byte ranges, and then save and display the corrupted image.
Go to:
Previous: Python program to convert list of integers to bytearray.
Next: Convert hexadecimal string to bytes.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.