Python program to convert list of integers to bytearray
Python Bytes and Byte Arrays Data Type: Exercise-3 with Solution
Write a Python program to create a bytearray from a given list of integers.
Sample Solution:
Code:
def bytearray_from_list(int_list):
byte_array = bytearray(int_list)
return byte_array
def main():
try:
nums = [72, 123, 21, 108, 222, 67, 44, 38, 10]
byte_array_result = bytearray_from_list(nums)
print("Integer List:", nums)
print("Bytearray:", byte_array_result)
print("Decoded String:", byte_array_result.decode("utf-8"))
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Integer List: [72, 123, 21, 108, 222, 67, 44, 38, 10] Bytearray: bytearray(b'H{\x15l\xdeC,&\n') An error occurred: 'utf-8' codec can't decode byte 0xde in position 4: invalid continuation byte
Flowchart:
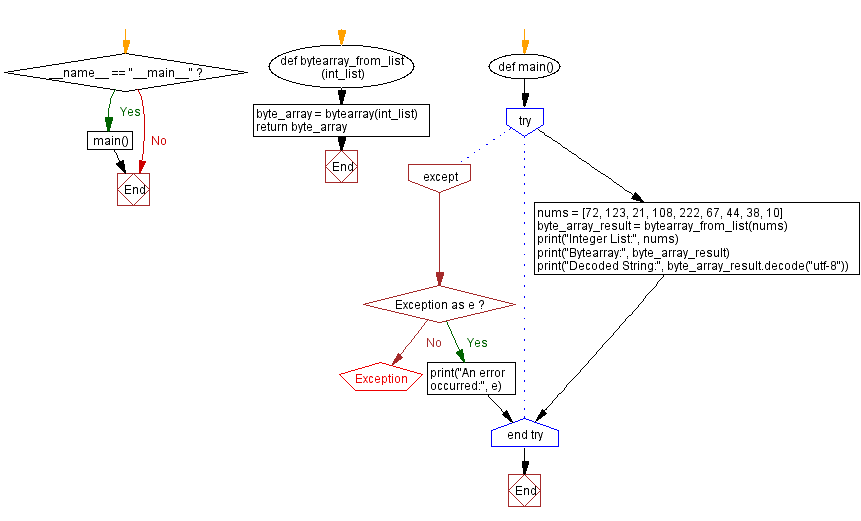
Previous: Python program for concatenating bytes objects.
Next: Python Program: Read and modify image as bytes.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/extended-data-types/python-extended-data-types-bytes-bytearrays-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics