Python program for concatenating bytes objects
Python Bytes and Byte Arrays Data Type: Exercise-2 with Solution
Write a Python program that concatenates two given bytes objects.
Sample Solution:
Code:
def concatenate_bytes(byte_obj1, byte_obj2):
concatenated_bytes = byte_obj1 + byte_obj2
return concatenated_bytes
def main():
try:
str_bytes1 = b"Python "
str_bytes2 = b"Exercises!"
concatenated_result = concatenate_bytes(str_bytes1, str_bytes2)
print("Bytes 1:", str_bytes1)
print("Bytes 2:", str_bytes2)
print("Concatenated Bytes:", concatenated_result)
print("Concatenated String:", concatenated_result.decode("utf-8"))
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Bytes 1: b'Python ' Bytes 2: b'Exercises!' Concatenated Bytes: b'Python Exercises!' Concatenated String: Python Exercises!
In the exercise above, the "concatenate_bytes()" function takes two bytes objects as input and concatenates them using the + operator. The "main()" function demonstrates the usage of this function by providing two sample bytes objects and printing the concatenated bytes and the concatenated string after decoding.
Flowchart:
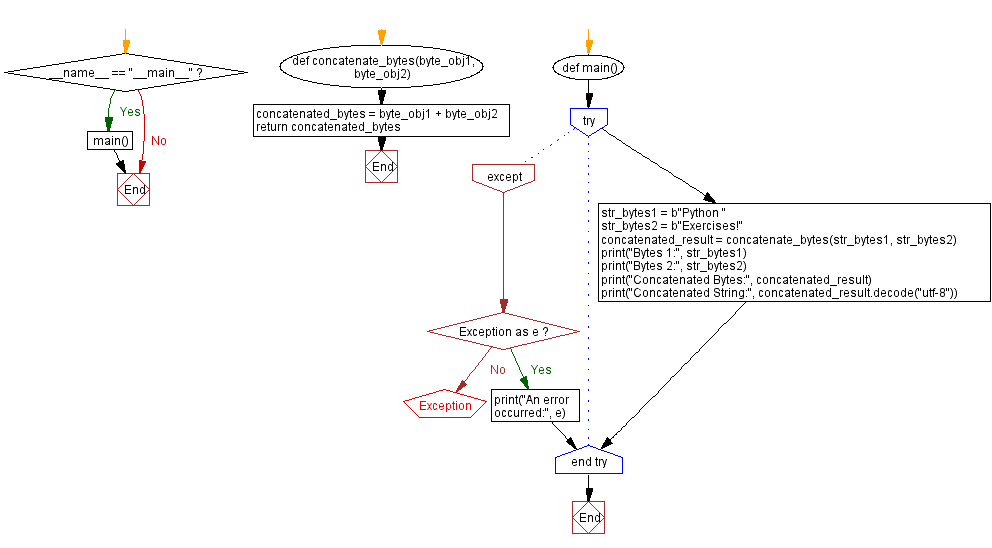
Previous: Python program to convert string to bytes using different Encodings.
Next: Python program to convert list of integers to bytearray.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/extended-data-types/python-extended-data-types-bytes-bytearrays-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics