Python Armstrong number generator: Generate next Armstrong numbers
14. Next Armstrong Number Generator
Write a Python program to implement a generator that generates the next Armstrong number after a given number.
When the sum of the cube of the individual digits of a number is equal to that number, the number is called Armstrong number. For Example 153 is an Armstrong number because 153 = 13+53+33.
Sample Solution:
Python Code:
def is_armstrong_number(n):
num_str = str(n)
num_len = len(num_str)
sum_of_cubes = sum(int(digit) ** num_len for digit in num_str)
return n == sum_of_cubes
def next_armstrong_number(start):
while True:
start += 1
if is_armstrong_number(start):
yield start
# Accept input from the user
n = int(input("Input a number: "))
# Create the generator object
armstrong_gen = next_armstrong_number(n)
# Generate and print the next Armstrong number
print("Next Armstrong number:")
for _ in range(1):
print(next(armstrong_gen))
Sample Output:
Input a number: 370 Next Armstrong number: 371
Enter a number: 153 Next Armstrong numbers: 370
Input a number: 407 Next Armstrong number: 1634
Explanation:
In the above exercise -
The is_armstrong_number() function first checks whether a given number is an Armstrong number. The function calculates the sum of cubes of digits and compares it with the original number to determine if it's an Armstrong number.
Next, the generator function next_armstrong_number() generates the next Armstrong numbers one by one.
Flowchart:
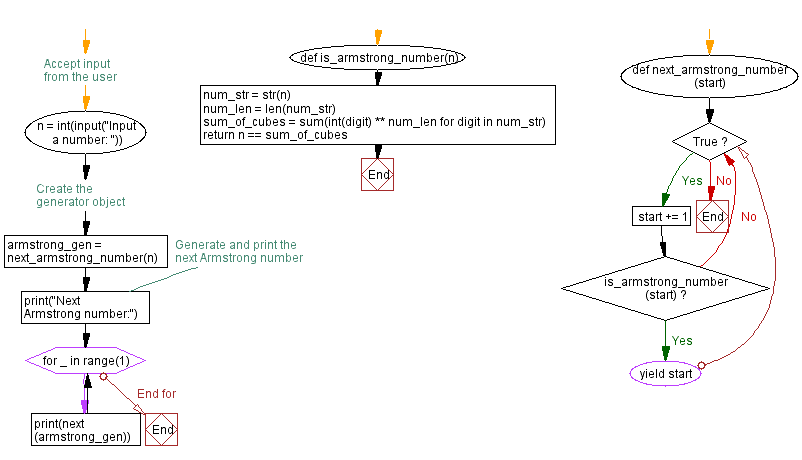
For more Practice: Solve these Related Problems:
- Write a Python program to create a generator that yields the next Armstrong number after a given number.
- Write a Python function that takes an integer and uses a generator to find and print the next Armstrong number.
- Write a Python script to yield the next two Armstrong numbers after a specified value and print them in a list.
- Write a Python program to implement a generator that finds Armstrong numbers in a given range and then outputs the first Armstrong number encountered after the input.
Go to:
Previous: Generate square, cube roots of numbers.
Next: Generate factors for a number.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.