Python Factor Generator: Generate factors for a number
15. Factors Generator
Write a Python program to create a generator function that generates all factors of a given number.
Sample Solution:
Python Code:
def create_factors(n):
for i in range(1, n+1):
if n % i == 0:
yield i
# Accept input from the user
n = int(input("Input a number: "))
# Create the generator object
factors_gen = create_factors(n)
# Generate and print all factors
print("Factors of", n)
for factor in factors_gen:
print(factor, end = ", ")
Sample Output:
Input a number: 1000 Factors of 1000 1, 2, 4, 5, 8, 10, 20, 25, 40, 50, 100, 125, 200, 250, 500, 1000,
Input a number: 56 Factors of 56 1, 2, 4, 7, 8, 14, 28, 56,
Input a number: 97 Factors of 97 1, 97,
Explanation:
In the above exercise -
We define the generator function create_factors() that takes a number n as input. It iterates over the range from 1 to n+1 and checks if n is divisible by each number. If it is, it yields that number.
Next we accept input from the user to determine the number for which we want to find factors. We create the generator object factors_gen and use it to generate and print all factors of the given number.
Flowchart:
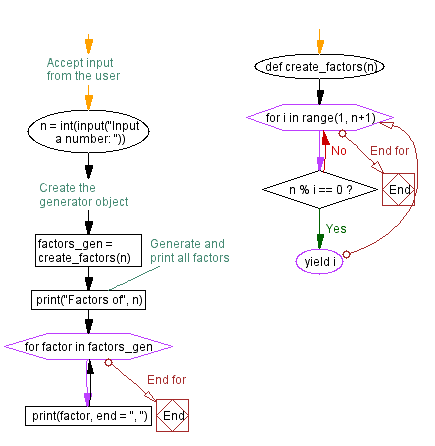
For more Practice: Solve these Related Problems:
- Write a Python program to create a generator that yields all factors of a given number, printing each factor as it is generated.
- Write a Python function that uses a generator to yield only the proper divisors of a number and then sums them.
- Write a Python script to generate factors of a number using a generator and compare the total number of factors with the expected count.
- Write a Python program to yield factors in ascending order and then output the list of factors and their product.
Go to:
Previous: Generate next Armstrong numbers.
Next: Calculate the average of a sequence.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.