Python prime factors generator using generators
9. Prime Factors Generator
Write a Python program that creates a generator that generates all prime factors of a given number.
Prime factorization of any given number is to breakdown the number into its factors until all of its factors are prime numbers. This can be achieved by dividing the given number from smallest prime number and continue it until all its factors are prime.
Sample Solution:
Python Code:
def is_prime(n):
# Check if the number is prime
if n < 2:
return False
for i in range(2, int(n ** 0.5) + 1):
if n % i == 0:
return False
return True
def prime_factors(n):
# Generate prime factors of a number
if n < 2:
return
for i in range(2, n + 1):
if n % i == 0 and is_prime(i):
yield i
# Accept input from the user
n = int(input("Input a number: "))
# Create the prime factors generator
factors_gen = prime_factors(n)
# Generate and print all prime factors
print("Prime factors of", n, "are:")
for factor in factors_gen:
print(factor)
Sample Output:
Input a number: 1729 Prime factors of 1729 are: 7 13 19
Input a number: 8763 Prime factors of 8763 are: 3 23 127
Input a number: 36 Prime factors of 36 are: 2 3
Explanation:
In the above exercise,
The "is_prime()" function checks whether a number is prime by iterating from 2 to the square root of the number and checking for any divisors.
The prime_factors generator function generates prime factors of a number by iterating from 2 to the given number. For each number, it checks if it is a factor of the input number and also a prime number using the is_prime function. If both conditions are met, it yields the prime factor.
The program accepts a number from the user and creates the prime_factors generator using that number. It iterates over the generator and prints all the prime factors.
Flowchart:
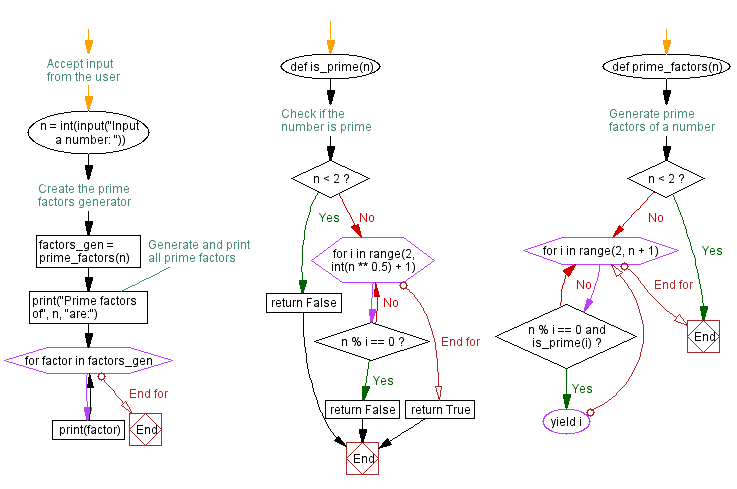
For more Practice: Solve these Related Problems:
- Write a Python program to create a generator that yields all prime factors of a given number one by one.
- Write a Python function that generates and prints the unique prime factors of a number using a generator.
- Write a Python script to use a generator to output prime factors in ascending order and then multiply them to verify the original number.
- Write a Python program to yield prime factors and then calculate their sum, printing the final sum.
Go to:
Previous: Python next palindrome number generator using generators.
Next: Python prime number generator using generators.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.