Python next palindrome number generator using generators
Python: Generators Yield Exercise-8 with Solution
Write a Python program that creates a generator function that generates the next palindrome number after a given number.
A palindromic number (also known as a numeral palindrome or a numeric palindrome) is a number (such as 16461) that remains the same when its digits are reversed. The first 30 palindromic numbers (in decimal) are: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 11, 22, 33, 44, 55, 66, 77, 88, 99, 101, 111, 121, 131, 141, 151, 161, 171, 181, 191, 202, ...
Sample Solution:
Python Code:
def is_palindrome(n):
# Check if the number is a palindrome
return str(n) == str(n)[::-1]
def next_palindrome(start):
# Start generating palindrome numbers from the next number
num = start + 1
while True:
if is_palindrome(num):
yield num
num += 1
# Accept input from the user
n = int(input("Input a number: "))
# Create the palindrome generator
palindrome_gen = next_palindrome(n)
# Find and print the next palindrome number
next_palindrome_num = next(palindrome_gen)
print("Next palindrome number after", n, "is:", next_palindrome_num)
Sample Output:
Input a number: 181 Next palindrome number after 181 is: 191
Input a number: 11 Next palindrome number after 11 is: 22
Input a number: 111 Next palindrome number after 111 is: 121
Explanation:
The "is_palindrome()" function checks whether a number is a palindrome by converting it to a string and comparing it with its reversed string.
The "next_palindrome()" generator function starts generating palindrome numbers from the next number after the given start number. It continuously increments num and checks whether it is a palindrome using the "is_palindrome()" function. If it is a palindrome, it yields the number.
The program accepts a number from the user and creates the next_palindrome generator starting from that number. It retrieves the next palindrome number using the next function on the generator, and then prints it.
Flowchart:
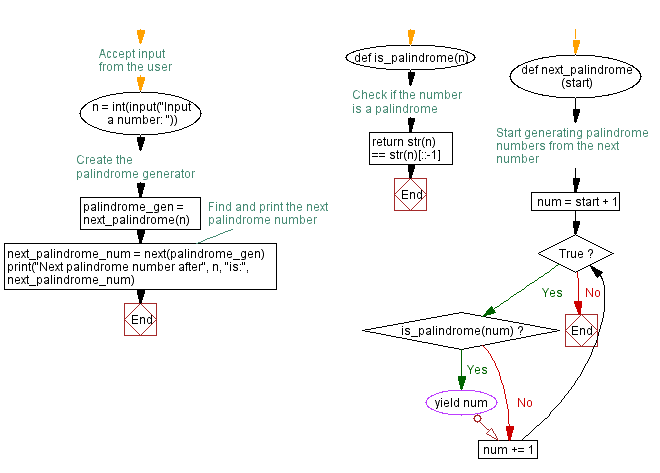
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Python Collatz sequence generator using generators.
Next: Python prime factors generator using generators.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics