Python: Find all index positions of the maximum and minimum values in a given list
Index of Max and Min Values in List
Write a Python program to find all index positions of the maximum and minimum values in a given list of numbers.
Sample Solution:
Python Code:
# Define a function 'position_max_min' that finds the index positions of the maximum and minimum values in a list
def position_max_min(nums):
# Find the maximum and minimum values in the 'nums' list
max_val = max(nums)
min_val = min(nums)
# Create a list 'max_result' with the index positions of the maximum value in 'nums'
max_result = [i for i, j in enumerate(nums) if j == max_val]
# Create a list 'min_result' with the index positions of the minimum value in 'nums'
min_result = [i for i, j in enumerate(nums) if j == min_val]
# Return both 'max_result' and 'min_result'
return max_result, min_result
# Create a list 'nums' with numeric values
nums = [12, 33, 23, 10, 67, 89, 45, 667, 23, 12, 11, 10, 54]
# Print a message indicating the original list
print("Original list:")
# Print the contents of 'nums'
print(nums)
# Call the 'position_max_min' function with 'nums' and store the result in 'result'
result = position_max_min(nums)
# Print a message indicating the index positions of the maximum value in the list
print("\nIndex positions of the maximum value of the said list:")
# Print the index positions of the maximum value from 'result'
print(result[0])
# Print a message indicating the index positions of the minimum value in the list
print("\nIndex positions of the minimum value of the said list:")
# Print the index positions of the minimum value from 'result'
print(result[1])
Sample Output:
Original list: [12, 33, 23, 10, 67, 89, 45, 667, 23, 12, 11, 10, 54] Index positions of the maximum value of the said list: [7] Index positions of the minimum value of the said list: [3, 11]
Flowchart:
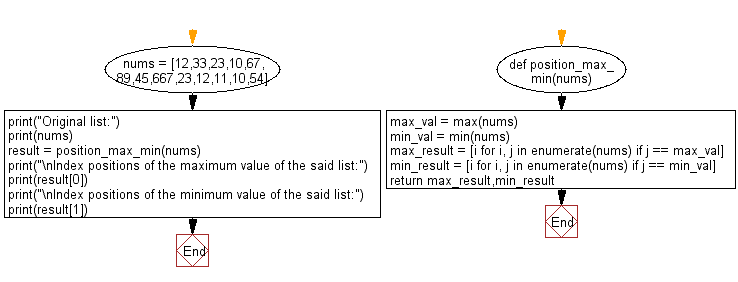
For more Practice: Solve these Related Problems:
- Write a Python program to find the second highest and lowest values in a list.
- Write a Python program to get the index of all peak values in a list.
- Write a Python program to locate positions of outliers in a list.
- Write a Python program to determine the range between max and min values.
Go to:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to count the same pair in three given lists.
Python Code Editor:
Next: Write a Python program to check common elements between two given list are in same order or not.What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.