Python: Unique values in a given list of lists
Python List: Exercise - 183 with Solution
Write a Python program to get the unique values in a given list of lists.
Visual Presentation:
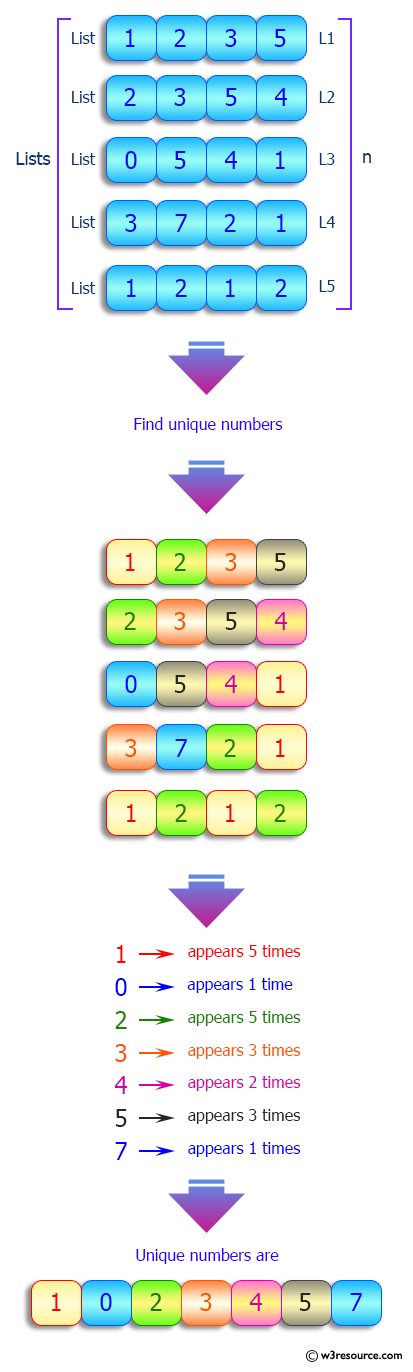
Sample Solution:
Python Code:
# Define a function called 'unique_values_in_list_of_lists' that extracts unique values from a list of lists.
def unique_values_in_list_of_lists(lst):
result = set(x for l in lst for x in l) # Flatten the list of lists and create a set to remove duplicates.
return list(result) # Convert the set back to a list.
# Create a list of lists 'nums', where each sublist contains integers.
nums = [[1, 2, 3, 5], [2, 3, 5, 4], [0, 5, 4, 1], [3, 7, 2, 1], [1, 2, 1, 2]]
# Print a message indicating the original list of lists.
print("Original list:")
print(nums)
# Call the 'unique_values_in_list_of_lists' function with 'nums' and store the unique values.
print("Unique values of the said list of lists:")
# Print the unique values extracted from the list of lists.
print(unique_values_in_list_of_lists(nums))
# Create a list of lists 'chars', where each sublist contains characters.
chars = [['h', 'g', 'l', 'k'], ['a', 'b', 'd', 'e', 'c'], ['j', 'i', 'y'], ['n', 'b', 'v', 'c'], ['x', 'z']]
# Print a message indicating the original list of lists.
print("\nOriginal list:")
print(chars)
# Call the 'unique_values_in_list_of_lists' function with 'chars' and store the unique values.
print("Unique values of the said list of lists:")
# Print the unique values extracted from the list of lists.
print(unique_values_in_list_of_lists(chars))
Sample Output:
Original list: [[1, 2, 3, 5], [2, 3, 5, 4], [0, 5, 4, 1], [3, 7, 2, 1], [1, 2, 1, 2]] Unique values of the said list of lists: [0, 1, 2, 3, 4, 5, 7] Original list: [['h', 'g', 'l', 'k'], ['a', 'b', 'd', 'e', 'c'], ['j', 'i', 'y'], ['n', 'b', 'v', 'c'], ['x', 'z']] Unique values of the said list of lists: ['e', 'd', 'c', 'b', 'x', 'k', 'n', 'h', 'g', 'j', 'i', 'a', 'l', 'y', 'v', 'z']
Flowchart:
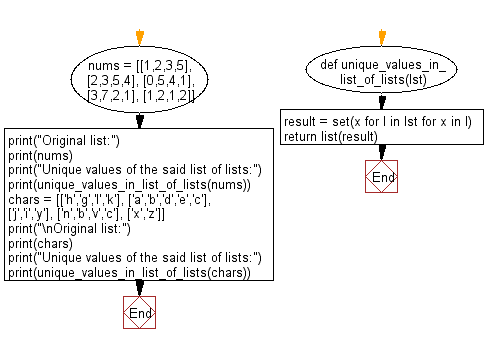
Python Code Editor:
Previous: Write a Python program to calculate the maximum and minimum sum of a sublist in a given list of lists.
Next: Write a Python program to form Bigrams of words in a given list of strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics