Python: Bigrams of words in a given list of strings
Python List: Exercise - 184 with Solution
Write a Python program to generate Bigrams of words from a given list of strings.
From Wikipedia:
A bigram or digram is a sequence of two adjacent elements from a string of tokens, which are typically letters, syllables, or words. A bigram is an n-gram for n=2. The frequency distribution of every bigram in a string is commonly used for simple statistical analysis of text in many applications, including in computational linguistics, cryptography, speech recognition, and so on.
Sample Solution:
Python Code:
# Define a function called 'bigram_sequence' that generates bigrams from a list of text sequences.
def bigram_sequence(text_lst):
result = [a for ls in text_lst for a in zip(ls.split(" ")[:-1], ls.split(" ")[1:])]
# Split each text sequence into words, generate bigrams, and flatten the list of bigrams.
return result
# Create a list of text sequences 'text'.
text = ["Sum all the items in a list", "Find the second smallest number in a list"]
# Print a message indicating the original list of text sequences.
print("Original list:")
print(text)
# Call the 'bigram_sequence' function with 'text' and store the bigrams.
print("\nBigram sequence of the said list:")
# Print the list of bigrams generated from the text sequences.
print(bigram_sequence(text))
Sample Output:
Original list: ['Sum all the items in a list', 'Find the second smallest number in a list'] Bigram sequence of the said list: [('Sum', 'all'), ('all', 'the'), ('the', 'items'), ('items', 'in'), ('in', 'a'), ('a', 'list'), ('Find', 'the'), ('the', 'second'), ('second', 'smallest'), ('smallest', 'number'), ('number', 'in'), ('in', 'a'), ('a', 'list')]
Flowchart:
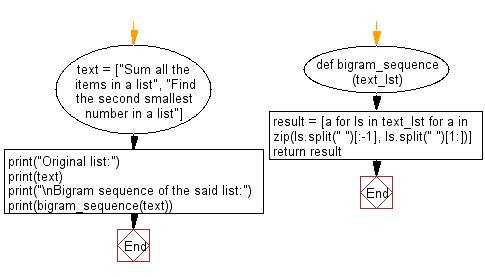
Python Code Editor:
Previous: Write a Python program to get the unique values in a given list of lists.
Next: Write a Python program to convert a given decimal number to binary list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics